In the blog post we are going to explain the student management system in java with database. And also explain the crud operations, registration form with database.
Managing student information efficiently is crucial for educational institutions. A Student Management System (SMS) helps schools, colleges, and universities maintain records of students, courses, grades, and more. One essential component of an SMS is a registration system that allows administrators to add new students to the database.
Prerequisites
Before diving into the coding part, ensure you have the following:
- Java Development Kit (JDK)
- MySQL Database
- MySQL JDBC Driver
Setting Up the Project
- Java Project Setup
- Create a new Java project in your favorite IDE and add the MySQL JDBC driver to your project’s class path.
- Database Setup
- First, let’s create a database and a table to store our registration data
CREATE DATABASE university;
USE university;
CREATE TABLE registrationForm (
first_name VARCHAR(50),
last_name VARCHAR(50),
email VARCHAR(50),
gender VARCHAR(10),
roll_no INT PRIMARY KEY
);
How to connect java with database?
To connect Java with a database using JDBC (Java Database Connectivity), you need to follow these steps:
- Choose a JDBC Driver: Select the appropriate JDBC driver for your database.
- Include the JDBC Driver in Your Project: Add the driver JAR file to your project’s classpath or specify it as a dependency in your project configuration file.
- Load the JDBC Driver: Use
Class.forName()
to load the JDBC driver class into memory. - Establish a Connection: Use
DriverManager.getConnection()
to establish a connection to the database, providing the JDBC URL and authentication credentials. - Create a Statement: Create a
Statement
orPreparedStatement
object to execute SQL queries. - Execute SQL Queries: Use
executeQuery()
to execute SELECT queries orexecuteUpdate()
for INSERT, UPDATE, and DELETE queries. - Process the Results: If executing a SELECT query, process the results using a
ResultSet
object. - Close the Connection: Close the
Connection
,Statement
, andResultSet
objects to release resources.
// Perform database insertion here
try {
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/university", "root", "password");
PreparedStatement ps = connection.prepareStatement("SELECT * FROM university.registrationform");
ResultSet rs = ps.executeQuery();
ps = connection.prepareStatement("INSERT INTO university.registrationform VALUES(?,?,?,?,?);");
ps.setString(1,firstName);
ps.setString(2,lastName);
ps.setString(3,email);
ps.setString(4,gender);
ps.setInt(5,roll_no);
ps.execute();
JOptionPane.showMessageDialog(this, "Registration Successful!");
connection.close();
} catch (SQLException ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage());
}
}
Source Code
Before download code please make sure to disable AD-BLOCKER because the ad revenue is used for hosting our Website. The download will automatically starts after 10 seconds of stay on redirected page.
Download Codewhat are crud operations?
CRUD operations stand for Create, Read, Update, and Delete. These are the basic functions that are typically associated with persistent storage in a database or any other data storage system. Here’s a brief explanation of each:
- Create: This operation involves the creation of new records or entries in a database. For example, adding a new user to a user database, inserting a new product into an inventory database, or creating a new document in a student management system.

- Read: The read operation involves retrieving or accessing existing data from the database. This can include fetching individual records, querying data based on certain criteria, or simply displaying data to users. Reading operations do not modify the data; they only retrieve it.

- Delete: This operation involves removing data from the database. It could mean deleting an entire record, removing specific fields from a record, or deleting records that meet certain criteria.
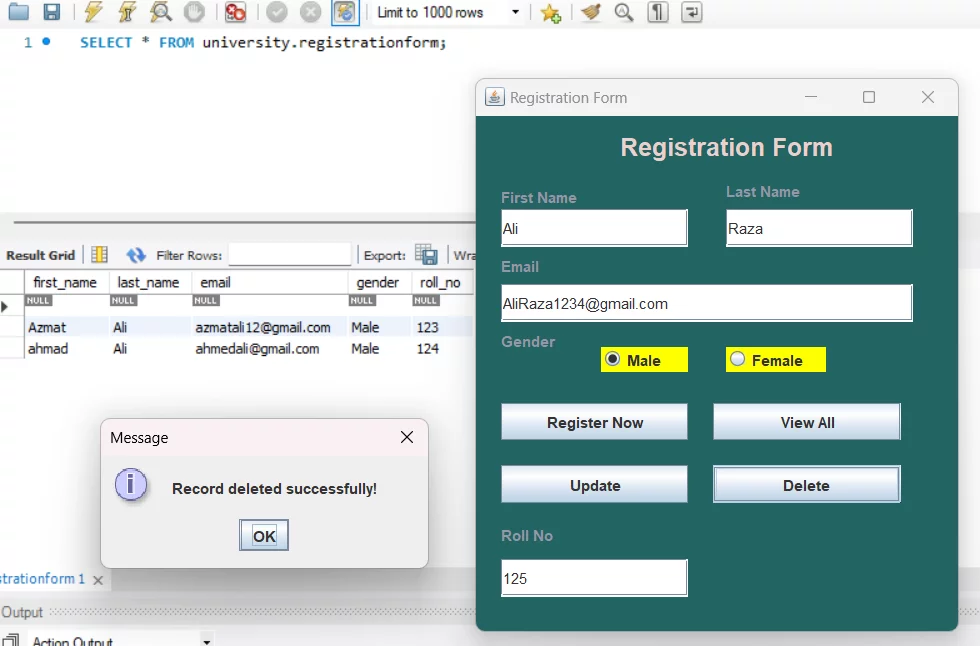
- Get the Project File of student management system in java with database from GitHub.
Key Features
1. Interface of Registration Form with database
The application presents users with a registration form interface featuring fields for first name, last name, email, gender, and roll number. Users can input their information seamlessly using text fields and radio buttons provided.
2. Intuitive Navigation
Navigation within the application is made simple with clearly labeled buttons for registration, viewing all records, updating records, and deleting records. These buttons are strategically placed for easy access and visibility.
3. Database Integration
The application seamlessly integrates with a MySQL database using JDBC (Java Database Connectivity). This allows for efficient storage and retrieval of user registration data, ensuring data persistence and reliability.
4. Event Handling
Event listeners are implemented to handle user interactions with the application. Actions such as registering a new user, viewing all records, updating existing records, and deleting records are performed based on user input, providing a responsive user experience.
How the registration form with database Works
The application’s functionality of registration form with database revolves around the RegistrationForm
class, which extends JFrame
to create the main application window. GUI components such as text fields, radio buttons, and buttons are initialized and arranged within a JPanel. Action listeners are attached to buttons to trigger specific operations such as registration, viewing, updating, and deleting records.
Database operations are encapsulated within methods like register()
, viewAll()
, update()
, and delete()
. These methods execute SQL queries to interact with the MySQL database, performing CRUD operations seamlessly.
Source code of student management system in java with database
The source code of student management system in java with database is given with complete connection with workbench but you only need to change the password or database name.
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.*;
class RegistrationForm extends JFrame {
private JTextField firstNameField, lastNameField, emailField;
private JRadioButton maleRadioButton, femaleRadioButton;
private JButton registerButton, viewAllButton, updateButton, deleteButton ;
private JTextField rollNoField;
public RegistrationForm() {
setTitle("Registration Form");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 450);
setLocationRelativeTo(null);
JPanel panel = new JPanel();
panel.setBackground(new Color(35, 100, 98));
panel.setLayout(null);
add(panel);
JLabel titleLabel = new JLabel("Registration Form");
titleLabel.setForeground(new Color(235, 208, 206));
titleLabel.setFont(new Font("Arial", Font.CENTER_BASELINE, 20));
titleLabel.setHorizontalAlignment(JLabel.CENTER);
titleLabel.setBounds(100, 10, 200, 30);
panel.add(titleLabel);
// First Name
JLabel firstNameLabel = new JLabel("First Name");
firstNameLabel.setForeground(new Color(149, 151, 166));
firstNameLabel.setBounds(20, 50, 100, 30);
panel.add(firstNameLabel);
firstNameField = new JTextField();
firstNameField.setBounds(20, 75, 150, 30);
panel.add(firstNameField);
// Last Name
JLabel lastNameLabel = new JLabel("Last Name");
lastNameLabel.setForeground(new Color(149, 151, 166));
lastNameLabel.setBounds(200, 50, 100, 20);
panel.add(lastNameLabel);
lastNameField = new JTextField();
lastNameField.setBounds(200, 75, 150, 30);
panel.add(lastNameField);
// Email
JLabel emailLabel = new JLabel("Email");
emailLabel.setForeground(new Color(149, 151, 166));
emailLabel.setBounds(20, 110, 100, 20);
panel.add(emailLabel);
emailField = new JTextField();
emailField.setBounds(20, 135, 330, 30);
panel.add(emailField);
// Gender
JLabel genderLabel = new JLabel("Gender");
genderLabel.setForeground(new Color(149, 151, 166));
genderLabel.setBounds(20, 170, 100, 20);
panel.add(genderLabel);
JLabel rollNoLabel = new JLabel("Roll No");
rollNoLabel.setForeground(new Color(149, 151, 166));
rollNoLabel.setBounds(20, 320, 100, 30);
panel.add(rollNoLabel);
rollNoField = new JTextField();
rollNoField.setBounds(20, 355, 150, 30);
panel.add(rollNoField);
maleRadioButton = new JRadioButton("Male");
maleRadioButton.setBackground(Color.yellow);
femaleRadioButton = new JRadioButton("Female");
femaleRadioButton.setBackground(Color.yellow);
maleRadioButton.setBounds(100, 185, 70, 20);
femaleRadioButton.setBounds(200, 185, 80, 20);
ButtonGroup genderGroup = new ButtonGroup();
genderGroup.add(maleRadioButton);
genderGroup.add(femaleRadioButton);
panel.add(maleRadioButton);
panel.add(femaleRadioButton);
// Register Button
registerButton = new JButton("Register Now");
registerButton.setBounds(20, 230, 150, 30);
panel.add(registerButton);
// View All Button
viewAllButton = new JButton("View All");
viewAllButton.setBounds(190, 230, 150, 30);
panel.add(viewAllButton);
// Update Button
updateButton = new JButton("Update");
updateButton.setBounds(20, 280, 150, 30);
panel.add(updateButton);
// Delete Button
deleteButton = new JButton("Delete");
deleteButton.setBounds(190, 280, 150, 30);
panel.add(deleteButton);
// Action Listeners
registerButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
register();
}
});
viewAllButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
viewAll();
}
});
updateButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
update();
}
});
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
delete();
}
});
setVisible(true);
}
private void register() {
// Perform registration operation and save data to the database
String firstName = firstNameField.getText();
String lastName = lastNameField.getText();
String email = emailField.getText();
String gender = maleRadioButton.isSelected() ? "Male" : "Female";
int roll_no = Integer.parseInt(rollNoField.getText());
// Perform database insertion here
try {
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/university", "root", "password");
PreparedStatement ps = connection.prepareStatement("SELECT * FROM university.registrationform");
ResultSet rs = ps.executeQuery();
ps = connection.prepareStatement("INSERT INTO university.registrationform VALUES(?,?,?,?,?);");
ps.setString(1,firstName);
ps.setString(2,lastName);
ps.setString(3,email);
ps.setString(4,gender);
ps.setInt(5,roll_no);
ps.execute();
JOptionPane.showMessageDialog(this, "Registration Successful!");
connection.close();
} catch (SQLException ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage());
}
}
private void viewAll() {
// Retrieve all records from the database and display them in the console
try {
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/university", "root", "password");
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM registrationForm");
System.out.println("All Records:");
while (resultSet.next()) {
String firstName = resultSet.getString("first_name");
String lastName = resultSet.getString("last_name");
String email = resultSet.getString("email");
String gender = resultSet.getString("gender");
int roll_no = resultSet.getInt("roll_no");
System.out.println("Name: " + firstName + " " + lastName + ", Email: " + email + ", Gender: " + gender + ", Roll No: " + roll_no);
}
connection.close();
} catch (SQLException ex) {
System.out.println("Error: " + ex.getMessage());
}
}
private void update() {
// Perform update operation on a selected record
String firstName = firstNameField.getText();
String lastName = lastNameField.getText();
String email = emailField.getText();
String gender = maleRadioButton.isSelected() ? "Male" : "Female";
int rollno = Integer.parseInt(rollNoField.getText());
// You can modify this query based on your database schema
String updateQuery = "UPDATE registrationForm SET first_name=?, last_name=?, email=?, gender=? WHERE roll_no=?";
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/university", "root", "password");
PreparedStatement preparedStatement = connection.prepareStatement(updateQuery)) {
// Set parameters for the prepared statement
preparedStatement.setString(1, firstName);
preparedStatement.setString(2, lastName);
preparedStatement.setString(3, email);
preparedStatement.setString(4, gender);
preparedStatement.setInt(5, rollno);
// Execute the update query
int rowsAffected = preparedStatement.executeUpdate();
if (rowsAffected > 0) {
JOptionPane.showMessageDialog(this, "Record updated successfully!");
} else {
JOptionPane.showMessageDialog(this, "No record found with the specified roll number.");
}
} catch (SQLException ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage());
}
}
private void delete() {
// Perform delete operation on a selected record
String rollno = rollNoField.getText();
// You can modify this query based on your database schema
String deleteQuery = "DELETE FROM registrationForm WHERE roll_no=?";
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/university", "root", "password");
PreparedStatement preparedStatement = connection.prepareStatement(deleteQuery)) {
// Set the roll number parameter for the prepared statement
preparedStatement.setString(1, rollno);
// Execute the delete query
int rowsAffected = preparedStatement.executeUpdate();
if (rowsAffected > 0) {
JOptionPane.showMessageDialog(this, "Record deleted successfully!");
} else {
JOptionPane.showMessageDialog(this, "No record found with the specified roll number.");
}
} catch (SQLException ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage());
}
}
public static void main(String[] args) {
RegistrationForm form = new RegistrationForm();
}
}
FAQ
What is Java Swing?
Java Swing is a set of GUI (Graphical User Interface) components provided by Java for building desktop applications. It offers a rich collection of widgets, layouts, and tools to create interactive and visually appealing user interfaces.
Why choose Java Swing for building desktop applications?
Java Swing offers several advantages for desktop application development, including platform independence, extensive documentation and community support, flexibility in customization, and compatibility with various operating systems.
Can Java Swing applications interact with databases?
Yes, Java Swing applications can interact with databases using JDBC (Java Database Connectivity). JDBC enables Java applications to execute SQL queries, perform CRUD operations (Create, Read, Update, Delete), and manage database connections seamlessly.
How does the user registration application store user data?
The user registration application stores user data in a MySQL database. JDBC is used to establish a connection to the database, execute SQL queries for data manipulation, and ensure data persistence and reliability
Conclusion
In conclusion, the registration form for student management system in java with database demonstrates the power and versatility of Java for developing desktop applications with rich graphical user interfaces. By leveraging Java Swing components and JDBC for database connectivity, developers can create intuitive and efficient applications that meet the needs of modern users. This will help you to know how to connect java with database? and what are crud operations.