In this blog post, we will explore how to build a basic Student Management System in C++ using GUI with wxWidgets. wxWidgets is a versatile library for creating cross-platform applications with a native look and feel. Our application will allow users to enter, save, search, delete, and display student data.
Managing student data efficiently is crucial for educational institutions. A well-designed software application can streamline the process, making data storage, retrieval, and manipulation tasks much easier.
Overview of the Application
The core of our application revolves around managing student information, which includes details like name, roll number, section, semester, and contact information.
We will use a structured approach by defining a STUDENT struct to hold our student data and a StudentManagementSystemFrame class that inherits from wxFrame to handle the GUI part of our application.
Key Components
- STUDENT Struct: Holds student data.
- StudentManagementSystemFrame Class: Manages the GUI and interacts with the user input.
- File Operations: Reads from and writes to a file for persistent storage.
- wxWidgets Controls: Define event handlers for buttons to perform actions like entering new data, displaying all students, searching for a specific student, deleting data, and exiting the application.
- Running the Application: To run the application, you need to have a wxWidgets application loop, which is provided by wxIMPLEMENT_APP(StudentManagementSystemApp);. This macro creates an entry point and initializes the application.
Step-by-Step Guide
Now we discuss step by step use of wxWidgets in detail.
What is wxWidgets?
Before we dive into the code, let’s understand what is wxWidgets. It is a library for C++ programming that lets developers create applications with graphical user interfaces (GUIs) that run on different operating systems like Windows, Mac, and Linux. Think of it as a toolkit that gives you components like buttons, text boxes, and windows to build your application’s interface without worrying about the underlying operating system.
Structuring Our Data with STUDENT
Syntax
struct STUDENT { wxString Name; wxString RollNo; wxString semester; wxString section; wxString contact; };
Each wxString is a type of string (a sequence of characters) used in wxWidgets to mange words and text.
Crafting of Main Window
The StudentManagementSystemFrame class is key to our program. It creates the main screen where users click around and do things. Because it’s a bigger version of wxFrame, it comes with all the tools and tricks of a regular wxWidgets window.
Setting the Stage: Initializing the Application
The adventure begins the moment you start the program. We get everything ready by putting in buttons for all kinds of tasks like adding info, showing info, looking for specific details, and getting rid of info. Here’s the gist of it:
- Adding Buttons: Think of making a dashboard where each button has its own job. We sprinkle our screen with a bunch of buttons for doing different stuff.
- Organizing the Layout: We use something called a “Sizer” in wxWidgets student management system in C++, which helps us arrange our buttons neatly in the window.
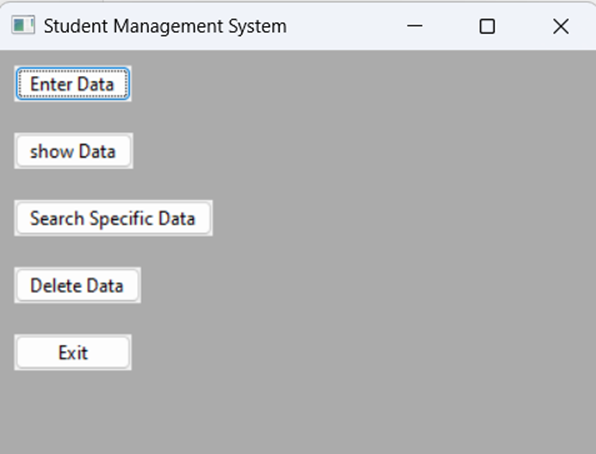
The Core Functionality
Let’s dive into the main features of our application:
Read Student Data
This process is similar to flipping open a book to read what’s inside. It finds and opens a document on your computer, goes through the student details saved there, and prepares it all for the program to work with.
Saving Student Data
Imagine it as writing down key points in your notebook to remember them later. Whenever you put in new details or change something about a student, this part makes sure to update that information in the document, so nothing gets lost after you close the program.
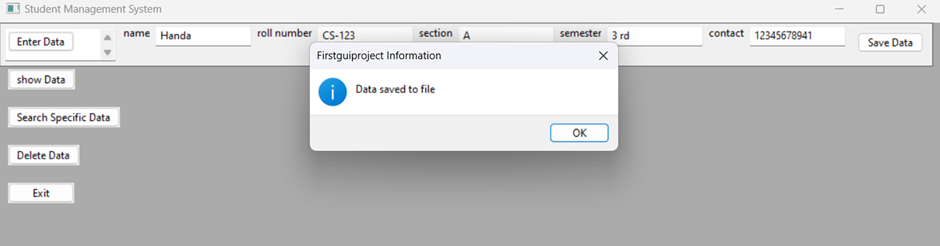
Adding New Student Information
Adding New Student Details Hitting the “Enter Data” button is similar to filling out a new sheet for each student. You type in their information into the spaces provided, and when you press “Save Data,” those details are kept both in the program and on your computer’s document.
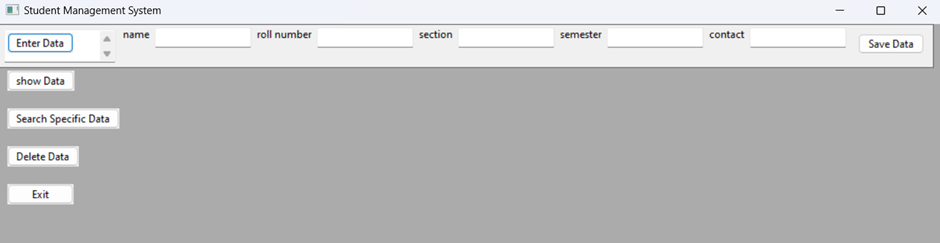
Displaying All Student Data
The “Show Data” button allows us to view all the student information we have stored, similar by clicking on details it will display a list or a table with all the entries.

Searching for a Specific Student
Sometimes, we need to find details about just one student. The “Search Specific Data” function lets us enter a student’s roll number, searches for it, and if found, displays the student’s details.

Deleting Student Information
We also have the capability to remove student data, either one at a time or all at once, depending on what we need. It’s like erasing an entry or an entire page from our notebook but with a safety step that asks us if we’re sure before it deletes anything. If you click on NO it will delete the specific data by entering roll number and if you click on YES it will delete all data that is saved in the file. It also show data after deleting the specific data from file.
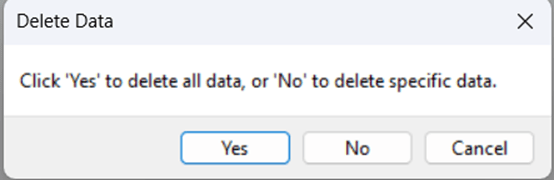
If you click on NO it will delete the specific data by entering roll number and if you click on YES it will delete all data that is saved in the file. It also show data after deleting the specific data from file.

It also show the remaining data after deleting the specific data of student from file.

Exiting the Application
Lastly, the “Exit” button serves as our way to close the application window, similar to turning off a device or closing a book.
Putting It All Together
Imagine building a small library system but for managing student data. When you run the application, it starts with an empty list. As you add, search, and manage data, it interacts with a file on your computer, reading and writing information as needed.
Conclusion
Making a program to student management system in C++ OOP shows how good wxWidgets is for creating programs with student management system in C++ using GUI. This work includes simple tasks like putting in, looking up, removing, and showing student details. It gives a strong base for making more advanced programs. By getting how this program is built and works, programmers can add more to it, like checking data is right, organizing, and picking out specific details, or even linking to a big database for more information.
Get the Project Files from GitHub
Download the project files from the GitHub repository via the provided link. You can clone the repository and utilize it on your local machine.
Check Out the Top 20 Final-Year Project Ideas for Beginners.
Code for Student Management System in C++ using GUI
#include <wx/wx.h>
#include <wx/button.h>
#include <wx/textctrl.h>
#include <wx/sizer.h>
#include <wx/statbmp.h>// // Include the header for image
#include <iostream>
#include <fstream>
#include <string>
#define SIZE 100
using namespace std;
struct STUDENT {
wxString Name;
wxString RollNo;
wxString semester;
wxString section;
wxString contact;
};
class StudentManagementSystemFrame : public wxFrame {
private:
//wxString dataValues[4];
public:
int CurrentTotalStudents;
STUDENT students[SIZE];
void ReadStudentData() {
// Implement logic to get stored data from the file
wxLogMessage("Get Stored Data");
string str;
int i = 0;
ifstream MyRead{ "C:\Temp\StudentManagement.txt" };
if (MyRead.is_open()) {
//getline(MyRead, str);
while (MyRead.peek() != EOF) {
/*while (!MyRead.eof()) {*/
getline(MyRead, str);
students[i].Name = wxString(str);
getline(MyRead, str);
students[i].RollNo = wxString(str);
getline(MyRead, str);
students[i].section = wxString(str);
getline(MyRead, str);
students[i].semester = wxString(str);
getline(MyRead, str);
students[i].contact = wxString(str);
i++;
}
CurrentTotalStudents = i;
}
else {
wxLogError("Error opening file for reading!");
}
MyRead.close();
}
void SaveStudentData() {
ofstream MyRecord{ "C:\Temp\StudentManagement.txt", ios::trunc }; // Open file in append mode
if (!MyRecord.is_open()) {
wxLogError("Error opening file for writing!");
return;
}
for (int i = 0; i < CurrentTotalStudents; ++i) {
MyRecord << students[i].Name << endl;
MyRecord << students[i].RollNo << endl;
MyRecord << students[i].section << endl;
MyRecord << students[i].semester << endl;
MyRecord << students[i].contact << endl;
}
MyRecord.close();
wxLogMessage("Data saved to file");
}
virtual bool OnInit() {
CurrentTotalStudents = 0;
return true;
}
StudentManagementSystemFrame(const wxString& title)
: wxFrame(NULL, wxID_ANY, title, wxDefaultPosition, wxSize(400, 300)) {
//
wxBoxSizer* mainSizer = new wxBoxSizer(wxVERTICAL);
wxButton* enterDataButton = new wxButton(this, wxID_ANY, "Enter Data");
enterDataButton->Bind(wxEVT_BUTTON, &StudentManagementSystemFrame::OnEnterData, this);
wxButton* loadDataButton = new wxButton(this, wxID_ANY, "show Data");
loadDataButton->Bind(wxEVT_BUTTON, &StudentManagementSystemFrame::onShowData, this);
wxButton* searchDataButton = new wxButton(this, wxID_ANY, "Search Specific Data");
searchDataButton->Bind(wxEVT_BUTTON, &StudentManagementSystemFrame::OnSearchSpecific, this);
wxButton* deleteDataButton = new wxButton(this, wxID_ANY, "Delete Data");
deleteDataButton->Bind(wxEVT_BUTTON, &StudentManagementSystemFrame::OnDeleteData, this);
wxButton* exitButton = new wxButton(this, wxID_ANY, "Exit");
exitButton->Bind(wxEVT_BUTTON, &StudentManagementSystemFrame::OnExit, this);
mainSizer->Add(enterDataButton, 0, wxALL, 10);
mainSizer->Add(loadDataButton, 0, wxALL, 10);
mainSizer->Add(searchDataButton, 0, wxALL, 10);
mainSizer->Add(deleteDataButton, 0, wxALL, 10);
mainSizer->Add(exitButton, 0, wxALL, 10);
SetSizer(mainSizer);
}
void OnEnterData(wxCommandEvent& event) {
// Create a panel with a specific size
wxPanel* panel = new wxPanel(this, wxID_ANY, wxDefaultPosition, wxSize(400, 200), wxTAB_TRAVERSAL | wxBORDER_SIMPLE);
wxBoxSizer* mainSizer = new wxBoxSizer(wxHORIZONTAL);
// Create a wxTextCtrl for displaying text
wxTextCtrl* outputTextCtrl = new wxTextCtrl(panel, wxID_ANY, wxEmptyString, wxDefaultPosition, wxDefaultSize, wxTE_MULTILINE | wxTE_READONLY);
mainSizer->Add(outputTextCtrl, 1, wxEXPAND | wxALL, 5);
// Create label and text controls
wxString labelLabels[] = { "name", "roll number", "section", "semester", "contact" };
wxString dataValues[4];// Array to store data from text boxes
for (int i = 0; i < 4; ++i) {
}
wxStaticText* label = new wxStaticText(panel, 100, labelLabels[0]);
mainSizer->Add(label, 0, wxALL, 3);
wxTextCtrl* textCtrl = new wxTextCtrl(panel, 101, wxEmptyString, wxDefaultPosition, wxDefaultSize, 0);
mainSizer->Add(textCtrl, 0, wxALL, 3);
label = new wxStaticText(panel, 102, labelLabels[1]);
mainSizer->Add(label, 0, wxALL, 3);
textCtrl = new wxTextCtrl(panel, 103, wxEmptyString, wxDefaultPosition, wxDefaultSize, 0);
mainSizer->Add(textCtrl, 0, wxALL, 3);
label = new wxStaticText(panel, 104, labelLabels[2]);
mainSizer->Add(label, 0, wxALL, 3);
textCtrl = new wxTextCtrl(panel, 105, wxEmptyString, wxDefaultPosition, wxDefaultSize, 0);
mainSizer->Add(textCtrl, 0, wxALL, 3);
label = new wxStaticText(panel, 106, labelLabels[3]);
mainSizer->Add(label, 0, wxALL, 3);
textCtrl = new wxTextCtrl(panel, 107, wxEmptyString, wxDefaultPosition, wxDefaultSize, 0);
mainSizer->Add(textCtrl, 0, wxALL, 3);
label = new wxStaticText(panel, 108, labelLabels[4]);
mainSizer->Add(label, 0, wxALL, 3);
textCtrl = new wxTextCtrl(panel, 109, wxEmptyString, wxDefaultPosition, wxDefaultSize, 0);
mainSizer->Add(textCtrl, 0, wxALL, 3);
wxButton* saveButton = new wxButton(panel, wxID_ANY, "Save Data");
saveButton->Bind(wxEVT_BUTTON, [this, dataValues](wxCommandEvent& event) {
wxTextCtrl* textCtrl = wxDynamicCast(FindWindowById(101), wxTextCtrl);
if (textCtrl) {
wxString textValue = textCtrl->GetValue();
students[CurrentTotalStudents].Name = textValue;
}
textCtrl = wxDynamicCast(FindWindowById(103), wxTextCtrl);
if (textCtrl) {
wxString textValue = textCtrl->GetValue();
students[CurrentTotalStudents].RollNo = textValue;
}
textCtrl = wxDynamicCast(FindWindowById(105), wxTextCtrl);
if (textCtrl) {
wxString textValue = textCtrl->GetValue();
students[CurrentTotalStudents].section = textValue;
}
textCtrl = wxDynamicCast(FindWindowById(107), wxTextCtrl);
if (textCtrl) {
wxString textValue = textCtrl->GetValue();
students[CurrentTotalStudents].semester = textValue;
}
textCtrl = wxDynamicCast(FindWindowById(109), wxTextCtrl);
if (textCtrl) {
wxString textValue = textCtrl->GetValue();
students[CurrentTotalStudents].contact = textValue;
}
CurrentTotalStudents++;
SaveStudentData();
});
mainSizer->Add(saveButton, 0, wxALL, 10);
panel->SetSizerAndFit(mainSizer);
}
void onShowData(wxCommandEvent& event) {
// Implement logic to load data from the file
wxLogMessage("Load Data Clicked");
ReadStudentData();
// Display loaded data (you can customize this part based on your GUI)
for (int i = 0; i < CurrentTotalStudents; i++) {
wxLogMessage("Student: %s, %s, %s, %s, %s",
students[i].Name, students[i].RollNo, students[i].semester, students[i].section, students[i].contact);
}
}
void OnSearchSpecific(wxCommandEvent& event) {
//wxLogMessage("Search Specific Data Clicked");
// Create a dialog to get the search criteria
wxTextEntryDialog dialog(this, "Enter the student's roll number:", "Search Specific Data");
if (dialog.ShowModal() == wxID_OK) {
wxString searchRollNo = dialog.GetValue();
// Read data from the file
ReadStudentData();
// Search for the student with the specified name
bool found = false;
for (int i = 0; i < CurrentTotalStudents; i++) {
if (students[i].RollNo == searchRollNo) {
// Display the found student's information
wxLogMessage("Student Found: %s, %s, %s, %s, %s",
students[i].Name, students[i].RollNo, students[i].semester, students[i].section, students[i].contact);
found = true;
break;
}
}
if (!found) {
wxLogMessage("Student with name '%s' not found.", searchRollNo);
}
}
}
void OnDeleteData(wxCommandEvent& event) {
wxMessageDialog* dialog = new wxMessageDialog(this, "Click 'Yes' to delete all data, or 'No' to delete specific data. ", "Delete Data", wxYES_NO | wxCANCEL | wxICON_QUESTION);
int result = dialog->ShowModal();
if (result == wxID_YES) {
// Delete all data
wxMessageDialog* confirmDialog = new wxMessageDialog(this, "Are you sure you want to delete all data?", "Confirm Deletion", wxYES_NO | wxICON_QUESTION);
int confirmResult = confirmDialog->ShowModal();
if (confirmResult == wxID_YES) {
// Delete all data from the file
std::ofstream clearFile("C:\Temp\StudentManagement.txt", std::ios::trunc);
clearFile.close();
// Reset total students count
CurrentTotalStudents = 0;
wxMessageBox("All data deleted successfully!", "Info", wxOK | wxICON_INFORMATION, this);
}
}
else if (result == wxID_NO) {
// Delete specific data
//wxString nameToDelete = wxGetTextFromUser("Enter Name to delete:", "Delete Specific Data", "", this);
wxString rollNoToDelete = wxGetTextFromUser("Enter Roll No to delete:", "Delete Specific Data", "", this);
// Read data from the file
ReadStudentData();
// Check if the entered Roll No is found in the data
bool found = false;
for (int i = 0; i < CurrentTotalStudents; ++i) {
if (students[i].RollNo == rollNoToDelete) {
// Shift data to remove the entry
for (int j = i; j < CurrentTotalStudents - 1; ++j) {
students[j] = students[j + 1];
}
--CurrentTotalStudents;
SaveStudentData();
wxMessageBox("Data deleted successfully!", "Info", wxOK | wxICON_INFORMATION, this);
found = true;
break;
}
}
if (!found) {
wxMessageBox("Student with the provided Roll No not found!", "Error", wxOK | wxICON_ERROR, this);
}
else {
// Display the remaining data after deletion
ReadStudentData();
// Display loaded data (you can customize this part based on your GUI)
for (int i = 0; i < CurrentTotalStudents; i++) {
wxLogMessage("Remaining Student: %s, %s, %s, %s, %s",
students[i].Name, students[i].RollNo, students[i].semester, students[i].section, students[i].contact);
}
}
}
}
void OnExit(wxCommandEvent& event) {
Close();
}
};
class StudentManagementSystemApp : public wxApp {
public:
virtual bool OnInit() {
StudentManagementSystemFrame* frame = new StudentManagementSystemFrame("Student Management System");
frame->Show(true);
return true;
}
};
wxIMPLEMENT_APP(StudentManagementSystemApp);
FAQS
How does wxWidgets support cross-platform GUI development?
wxWidgets provides a wide range of widgets (like buttons, text boxes, and windows) that make it easier to develop cross-platform applications.
How do you structure student data in the C++ Student Management System?
Student data is structured using a STUDENT
struct, which includes fields for the student’s name, roll number, semester, section, and contact information. This approach organizes the data systematically for easy management and access within the application.
Can you customize the GUI layout of the Student Management System in C++?
Yes, the GUI layout can be customized using wxWidgets’ Sizer functionality, which helps in arranging buttons and other widgets in a structured and visually appealing manner.
How do you run the Student Management System application?
To run the application, you need to compile the C++ code using a compiler that supports wxWidgets and then execute the compiled program. The application uses a wxWidgets application loop, initiated by the wxIMPLEMENT_APP(StudentManagementSystemApp);
macro, to start and manage the GUI.