In this code you will learn about how to create the registration form in java with MySQL. And the connection of IntelliJ with MySQL workbench.
Implementing the Login Form
We start by creating a login form GUI using Swing components such as JFrame
, JLabel
, JTextField
, JPasswordField
, and JButton
. These components provide the necessary user interface elements for inputting and submitting login credentials.
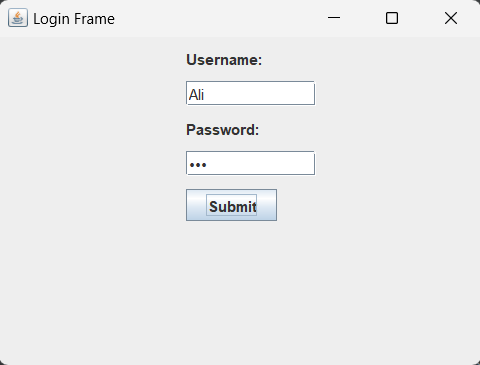
NOTE
Before running the code of java registration form using MySQL you have to change the password and user name like in this code the user name is “root” and the password is “password”.
- Get the Project Files from GitHub.
- You can also download its Zip File by clicking on Download button.
How to connect Java application with MySQL Workbench
Here are the general steps to connect your Java application with MySQL Workbench:
- Install MySQL Server: Make sure you have MySQL Server installed on your system. MySQL Workbench connects to a MySQL Server instance, so you need to have MySQL Server running to connect to it.
- Include JDBC Driver: Download the JDBC driver for MySQL, which is a JAR file. You can usually find it on the official MySQL website. Include this JAR file in your Java project’s classpath.
- Write Java Code: Write Java code to establish a connection to the MySQL database using JDBC. You’ll need to provide the database URL, username, and password to connect.
public class Main {
public static void main(String[] args) {
String URL= "jdbc:mysql://localhost:3306/university";
String username = "root";
String password = "your_password";
try {
// Establish connection
Connection connection = DriverManager.getConnection(url, username, password);
System.out.println("Connected to the database");
// Perform database operation
} catch (SQLException e) {
System.err.println("Error connecting to the database: " + e.getMessage());
}
}
}
Error handling in java login form
Error heandling in java login form can be detacted as:
- If the entered username and password match the expected values (e.g., “admin” for both username and password), a dialog with the message “Login successful” is displayed.
- If the entered username or password is incorrect, a dialog with the message “Invalid username or password” is displayed, along with an error icon (
JOptionPane.ERROR_MESSAGE
).
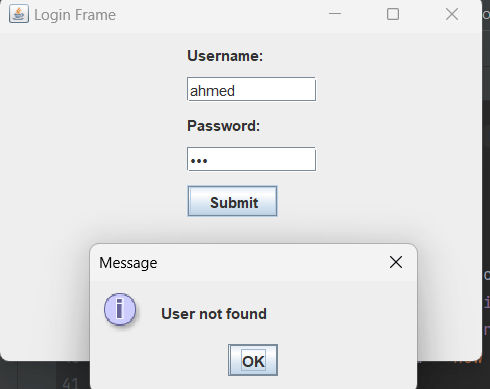
Source Code
Here is the code of registration form in java with MySQL. Remember one thing befor running this code you have to change the password and user name.
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.*;
class LoginForm implements ActionListener {
private JFrame frame;
private JLabel userNameLabel;
private JLabel passwordLabel;
private JTextField userField;
private JPasswordField passwordField;
private JButton submitButton;
public LoginForm() {
frame = new JFrame("Login Frame");
userNameLabel = new JLabel("Username: ");
passwordLabel = new JLabel("Password: ");
userField = new JTextField(10);
passwordField = new JPasswordField(10);
submitButton = new JButton("Submit");
submitButton.addActionListener(this);
frame.setLayout(new FlowLayout(FlowLayout.LEFT, 150, 10));
frame.add(userNameLabel);
frame.add(userField);
frame.add(passwordLabel);
frame.add(passwordField);
frame.add(submitButton);
frame.setSize(400, 300);
frame.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == submitButton) {
String username = userField.getText().trim();
String password = new String(passwordField.getPassword()).trim();
if (username.isEmpty() || password.isEmpty()) {
JOptionPane.showMessageDialog(null, "Please enter the username and password.");
return;
}
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/university", "root", "password");
PreparedStatement statement = connection.prepareStatement("SELECT * FROM student WHERE stuname = ?")) {
statement.setString(1, username);
ResultSet resultSet = statement.executeQuery();
if (resultSet.next()) {
String storedPassword = resultSet.getString("password");
// Check password using a secure method, e.g., bcrypt
if (password.equals(storedPassword)) {
JOptionPane.showMessageDialog(null, "Login successful");
} else {
JOptionPane.showMessageDialog(null, "Incorrect password");
}
} else {
JOptionPane.showMessageDialog(null, "User not found");
}
} catch (SQLException ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(null, "Error: " + ex.getMessage());
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(LoginForm::new);
}
}
FAQ
Why is error handling important in a Java login form?
Error handling in a Java login form is crucial for providing feedback to users when they enter incorrect credentials. It enhances user experience by guiding users through the authentication process and helps prevent frustration.
What libraries are used in this program?
This program uses javax.swing for GUI components and java.sql for database connectivity.
Conclusion of registration form in java with MySQL
Building a registration form in java with MySQL requires careful consideration of various factors, including password security, protection against SQL injection attacks, and proper resource management. By following best practices and leveraging the power of Java and MySQL, we can create robust and secure authentication systems that safeguard user data and ensure a seamless user experience.