Today we are going to study the prefix to infix C++ using stack. Convertors of Stacks like prefix to infix. Also the applications and principles of this conversion.
What are Prefix and Infix Expressions?
Before we dive into the conversion process, let’s clarify what prefix and infix expressions are:
- Prefix Expression: Also known as Polish notation, prefix notation requires operators to precede their operands. For example, the expression “+AB” represents the addition of operands A and B.
- Infix Expression: In infix notation, operators are placed between their operands. For instance, “A + B” is an infix expression where A and B are operands, and ‘+’ is the operator.
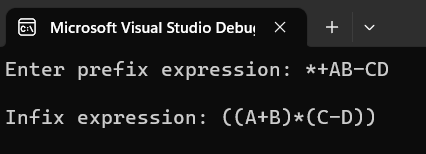
Conversion of prefix to infix in C++ using stack
Here is the key reasons of conversion of prefix to infix in C++ using stack is needed in Data Structures. Let’s break down the conversion process step by step:
- Start from the Right: In prefix notation, operators precede their operands. So, we start scanning the expression from right to left.
- Encounter Operators First: We encounter operators first in prefix notation. In this example, the operators are ‘*’ (multiplication) and ‘+’ (addition) followed by ‘-‘.
- Identify Operands: After encountering an operator, we identify the next two characters as operands. In this case, ‘A’ and ‘B’ are operands for the ‘+’ operator, and ‘C’ and ‘D’ are operands for the ‘-‘ operator.
- Combine Operands and Operator: For each operator, we combine the corresponding operands and the operator in infix notation. For example, for the ‘+’ operator, we form the expression “(A + B)” and for the ‘-‘ operator, we form “(C – D)”.
- Replace Original Expression: We replace the original expression with the infix expression formed in the previous step. So, “*+AB-CD” becomes “* (A + B) (C – D)”.
- Repeat Until Whole Expression is Converted: We continue this process until the entire expression is converted.
- Final Expression: After converting the whole expression, we get the final infix expression: “((A + B) * (C – D))”.
So, the prefix expression “*+AB-CD” is converted to the infix expression “((A + B) * (C – D))”.
NOTE
- Get the Project Files from GitHub.
Source Code
Before download code please make sure to disable AD-BLOCKER because the ad revenue is used for hosting our Website. The download will automatically starts after 10 seconds of stay on redirected page.
Download CodeApplications of prefix to infix
Here is the come applications of conversion of prefix to infix in C++ using stack
- Compiler Design: In compiler design, converting expressions between different notations is a common task. This conversion may occur during the parsing phase or as part of optimizations.
- Algorithm Design: Some algorithms, such as those used in symbolic computation or computer algebra systems, require manipulation of mathematical expressions. Converting prefix expressions to infix can be a part of such algorithms.
- Mathematical Software: Mathematical software often deals with complex expressions. Converting prefix expressions to infix can make the output more readable and understandable for users.
FAQ
What is prefix notation, and how does it differ from infix notation?
Prefix notation, also known as Polish notation, is a way of writing expressions in which the operators precede their operands.
Why would I need to converters in C++ using stack?
Converting prefix expressions to infix notation can be useful for various purposes, including expression evaluation, parsing, debugging, and better human readability. Infix notation is more intuitive and familiar to humans, making it easier to understand and work with.
What are some common applications of prefix to infix conversion?
Common applications include expression evaluation, mathematical software, compiler design, algorithm design, debugging, logging, and educational purposes in teaching expression manipulation and parsing algorithms.
Source Code of prefix to infix C++ using stack
here’s an implementation of conversion of prefix to infix in C++ using stack data structure:
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
string prefixToInfix(string prefix) {
stack<string> st;
for (int i = prefix.length() - 1; i >= 0; i--) {
if (isOperator(prefix[i])) {
string operand1 = st.top();
st.pop();
string operand2 = st.top();
st.pop();
string result = "(" + operand1 + prefix[i] + operand2 + ")";
st.push(result);
}
else {
string operand(1, prefix[i]);
st.push(operand);
}
}
return st.top();
}
int main() {
string prefixExpression;
cout << "Enter prefix expression: ";
cin >> prefixExpression;
string infixExpression = prefixToInfix(prefixExpression);
cout << endl;
cout << "Infix expression: " << infixExpression << endl;
return 0;
}
Conclusion
conversion of prefix to infix in C++ using stack can be useful in various applications, such as expression evaluation and syntax parsing. By understanding the conversion algorithm and implementing it in C++, you can manipulate arithmetic expressions in different notations effectively.