Notepad Project in Java with source code: We can easily create a Notepad using Java on the console and using the Graphic User Interface with the help of Java Swing and AWT. This article explores the features, functionality, and underlying Java code of the Console Notepad, empowering users to manage their notes effectively.
First, let’s have a look at the Features and source code for creating a Notepad in Java. You can also download the full project from the download button below and you can also clone our Github repository to start your coding.
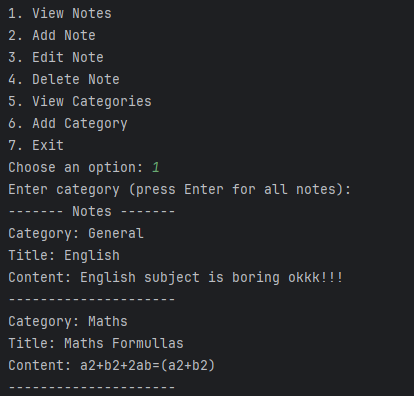
Features of Console Notepad
Feature | Description |
---|---|
View Notes | Easily peruse your notes, organized by categories. You can filter notes based on a specific category or view all notes at once. |
Add Note | Seamlessly add new notes to your notepad. The application prompts you for the category, title, and content of the note, ensuring a straightforward note-creation process. |
Edit Note | Effortlessly edit existing notes by specifying the title of the note you wish to modify. Update the content of the note with a new input, providing a quick way to refine your notes. |
Delete Note | Remove unwanted notes from your notepad. Enter the title of the note you want to delete, and the application efficiently handles the deletion process. |
View Categories | Get an overview of available categories, helping you organize your notes effectively. This feature facilitates easy categorization and retrieval of information. |
Add Category | Enhance organization by adding new categories. The application prompts you to enter the name of the new category, allowing for a dynamic and customizable categorization system. |
Exit | Gracefully exit the Console Notepad when you’re done, ensuring a smooth user experience. |
Export Notes (Not Available) | The Console Notepad currently does not have a built-in export feature. However, you can manually copy the content from the “notes.txt” file and paste it into a text document or any other application of your choice. |
Source Code
import java.io.*;
import java.util.*;
public class ConsoleNotepad {
private static final String FILE_PATH = "notes.txt";
private static final String CATEGORIES_FILE_PATH = "categories.txt";
private static final String DEFAULT_CATEGORY = "General";
public static void main(String[] args) {
initializeCategoriesFile();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("1. View Notes");
System.out.println("2. Add Note");
System.out.println("3. Edit Note");
System.out.println("4. Delete Note");
System.out.println("5. View Categories");
System.out.println("6. Add Category");
System.out.println("7. Exit");
System.out.print("Choose an option: ");
int choice = scanner.nextInt();
scanner.nextLine(); // Consume the newline character
switch (choice) {
case 1:
viewNotes();
break;
case 2:
addNote();
break;
case 3:
editNote();
break;
case 4:
deleteNote();
break;
case 5:
viewCategories();
break;
case 6:
addCategory();
break;
case 7:
System.out.println("Exiting Notepad. Goodbye!");
System.exit(0);
default:
System.out.println("Invalid choice. Please try again.");
}
}
}
private static void initializeCategoriesFile() {
try {
File categoriesFile = new File(CATEGORIES_FILE_PATH);
if (!categoriesFile.exists()) {
categoriesFile.createNewFile();
BufferedWriter writer = new BufferedWriter(new FileWriter(categoriesFile, true));
writer.write(DEFAULT_CATEGORY);
writer.newLine();
writer.close();
}
} catch (IOException e) {
System.out.println("Error initializing categories file. " + e.getMessage());
}
}
private static void viewNotes() {
System.out.print("Enter category (press Enter for all notes): ");
Scanner scanner = new Scanner(System.in);
String categoryFilter = scanner.nextLine().trim();
try (BufferedReader reader = new BufferedReader(new FileReader(FILE_PATH))) {
String line;
System.out.println("------- Notes -------");
while ((line = reader.readLine()) != null) {
String[] noteInfo = line.split("\\|", 3);
if (noteInfo.length >= 3) {
String noteCategory = noteInfo[0].trim();
String noteTitle = noteInfo[1].trim();
String noteContent = noteInfo[2].trim();
if (categoryFilter.isEmpty() || noteCategory.equals(categoryFilter)) {
System.out.println("Category: " + noteCategory);
System.out.println("Title: " + noteTitle);
System.out.println("Content: " + noteContent);
System.out.println("---------------------");
}
} else {
System.out.println("Invalid format in line: " + line);
}
}
} catch (IOException e) {
System.out.println("Error reading notes. " + e.getMessage());
}
}
private static void addNote() {
try {
System.out.print("Enter category (press Enter for default): ");
Scanner scanner = new Scanner(System.in);
String category = scanner.nextLine().trim();
if (category.isEmpty()) {
category = DEFAULT_CATEGORY;
}
BufferedWriter writer = new BufferedWriter(new FileWriter(FILE_PATH, true));
System.out.print("Enter note title: ");
String title = scanner.nextLine();
System.out.print("Enter note content: ");
String content = scanner.nextLine();
writer.write(category + " | " + title + " | " + content);
writer.newLine();
writer.close();
System.out.println("Note added successfully!");
} catch (IOException e) {
System.out.println("Error adding note. " + e.getMessage());
}
}
private static void editNote() {
System.out.print("Enter note title to edit: ");
Scanner scanner = new Scanner(System.in);
String titleToEdit = scanner.nextLine();
List<String> notes = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(FILE_PATH))) {
String line;
while ((line = reader.readLine()) != null) {
String[] noteInfo = line.split("\\|", 3);
if (noteInfo.length >= 3) {
String noteTitle = noteInfo[1].trim();
if (noteTitle.equals(titleToEdit)) {
System.out.print("Enter new content for the note: ");
String newContent = scanner.nextLine();
line = noteInfo[0].trim() + " | " + noteTitle + " | " + newContent.trim();
}
notes.add(line);
} else {
System.out.println("Invalid format in line: " + line);
}
}
} catch (IOException e) {
System.out.println("Error reading notes. " + e.getMessage());
}
try (BufferedWriter writer = new BufferedWriter(new FileWriter(FILE_PATH))) {
for (String note : notes) {
writer.write(note);
writer.newLine();
}
} catch (IOException e) {
System.out.println("Error writing notes. " + e.getMessage());
}
System.out.println("Note edited successfully!");
}
private static void deleteNote() {
System.out.print("Enter note title to delete: ");
Scanner scanner = new Scanner(System.in);
String titleToDelete = scanner.nextLine();
List<String> notes = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(FILE_PATH))) {
String line;
while ((line = reader.readLine()) != null) {
String noteTitle = line.split("\\|")[1].trim();
if (!noteTitle.equals(titleToDelete)) {
notes.add(line);
}
}
} catch (IOException e) {
System.out.println("Error reading notes. " + e.getMessage());
}
try (BufferedWriter writer = new BufferedWriter(new FileWriter(FILE_PATH))) {
for (String note : notes) {
writer.write(note);
writer.newLine();
}
} catch (IOException e) {
System.out.println("Error writing notes. " + e.getMessage());
}
System.out.println("Note deleted successfully!");
}
private static void viewCategories() {
try (BufferedReader reader = new BufferedReader(new FileReader(CATEGORIES_FILE_PATH))) {
String line;
System.out.println("------- Categories -------");
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
System.out.println("--------------------------");
} catch (IOException e) {
System.out.println("Error reading categories. " + e.getMessage());
}
}
private static void addCategory() {
try (BufferedWriter writer = new BufferedWriter(new FileWriter(CATEGORIES_FILE_PATH, true))) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter new category: ");
String newCategory = scanner.nextLine();
writer.write(newCategory);
writer.newLine();
System.out.println("Category added successfully!");
} catch (IOException e) {
System.out.println("Error adding category. " + e.getMessage());
}
}
}
GitHub Repository
You can visit our GitHub repository to explore more interesting projects and to clone the GitHub repository to use that project directly. Click on the GitHub Repository below to do so.
Download Full Notepad Project in Java
You can download the Notepad Project in Java through the Download Button Bellow.
How to Use Project
- View Notes: Enter the category name (press Enter for all notes) and get a structured view of your notes.
- Add Note: Input the category, note title, and content to add a new note to your notepad.
- Edit Note: Enter the note title you want to edit and provide new content for the note.
- Delete Note: Specify the note title to delete, and the application will handle the removal.
- View Categories: Explore existing categories and organize your notes effectively.
- Add Category: Introduce a new category for dynamic and customizable organization.
- Exit: Choose this option to gracefully exit the Console Notepad.
FAQ
How does the Console Notepad handle errors during input validation?
Errors during input validation are effectively handled by utilizing try-catch blocks to catch exceptions and provide appropriate error messages to the user. Additionally, the application uses loops to prompt the user for input until valid data is provided.
Can I customize categories in the Console Notepad?
Yes, the Console Notepad allows you to add new categories, providing flexibility and customization in organizing your notes.
What happens if I try to edit or delete a note that doesn’t exist?
If you attempt to edit or delete a note with a title that doesn’t exist, the application will notify you that the note is not found. It’s crucial to provide the correct note title for editing or deletion.
Is it possible to change the default category for new notes?
Yes, when adding a new note, you can specify the category. Press Enter to use the default category (“General”) or input a different category name.
Can I export my notes from the Console Notepad to other formats?
The Console Notepad currently does not have a built-in export feature. However, you can manually add the content from the “notes.txt” file and paste it into a text document or any other application of your choice.