Today we learn about Java CRUD operations like how to create, read, update, and delete data in a Java application, with file handling using an Inventory Management System as a practical example with our comprehensive guide.
What Are CRUD Operations?
CRUD operations stand for Create, Read, Update, and Delete. These operations represent the four basic functions that can be performed on data in a persistent storage system such as a database or file system.
Overview of Java
Java’s platform-independent nature, coupled with its robust standard libraries, makes it an ideal choice for developing applications requiring reliable database interactions. Its object-oriented approach simplifies complex concepts and enhances code reusability and maintenance.
Note
you can also get the git repository of its code.
you can also download its code from Zip file.
Setting Up the Environment
Before diving into CRUD operations, setting up a proper development environment is crucial. This section walks you through installing Java and your preferred Integrated Development Environment (IDE), such as Eclipse or IntelliJ IDEA, ensuring you have the necessary tools to begin coding.
CRUD Operations In Java

Creating Data
The first cornerstone of CRUD operations, the creation process, is exemplified by adding new items to the inventory. This section walks you through the implementation details, from user input to data storage.
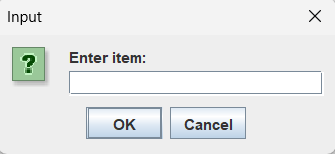
private void onEnterData() {
String item = JOptionPane.showInputDialog(this, "Enter item:");
if (item != null && !item.isEmpty()) {
inventory.add(item);
JOptionPane.showMessageDialog(this, "Item added.");
}
}
Reading Data
Reading data is crucial for visibility and management. Learn how to display the inventory items to the user, employing Java’s collection framework and GUI components for a seamless experience.

private void onShowData() {
displayArea.setText("");
for (String item : inventory) {
displayArea.append(item + "\n");
}
}
Updating Data
Updating existing data ensures the relevance and accuracy of the application’s information. This part delves into modifying items within the inventory, showcasing the necessary steps to locate, modify, and save the updated data.

private void onUpdateData() {
String currentData = JOptionPane.showInputDialog(this, "Enter old item:");
if (currentData != null && inventory.contains(currentData)) {
String newData = JOptionPane.showInputDialog(this, "Enter new item for " + currentData + ":");
if (newData != null && !newData.isEmpty()) {
inventory.set(inventory.indexOf(currentData), newData);
JOptionPane.showMessageDialog(this, "Item updated.");
}
} else {
JOptionPane.showMessageDialog(this, "Item not found.");
}
}
Deleting Data
The ability to delete data is as critical as creating it. This segment covers the deletion of items from the inventory, emphasizing user interaction and data integrity.
private void onDeleteData() {
String itemToDelete = JOptionPane.showInputDialog(this, "Enter item to delete:");
if (itemToDelete != null && inventory.contains(itemToDelete)) {
inventory.remove(itemToDelete);
JOptionPane.showMessageDialog(this, "Item deleted.");
} else {
JOptionPane.showMessageDialog(this, "Item not found.");
}
}
Creating the User Interface
A user-friendly interface is pivotal for any application. Discover how to design and implement an intuitive layout for the Inventory Management System, focusing on user input and feedback mechanisms.
Java file handling
Persistence is key to maintaining data between sessions. Explore how to save and load the inventory data from a file, ensuring your application’s data is persistent and reliable.
Conclusion
Reflecting on the key points, this guide underscores the significance of mastering Java CRUD operations. The Inventory Management System exemplifies their practical application, offering a comprehensive learning experience that bridges theory and practice.
Related Articles
FAQ
What is the best database for Java CRUD operations?
The best database for Java CRUD operations depends on the specific needs and goals of your project.
How do I secure my CRUD operations in Java?
Securing CRUD operations in Java involves several strategies to protect data integrity and prevent unauthorized access. Implementing strong authentication and authorization mechanisms is critical to ensure that only authenticated users can perform CRUD operations.
What are the challenges of implementing CRUD operations in Java?
Implementing CRUD operations in Java can present several challenges, including managing database connections efficiently, ensuring data consistency, handling exceptions gracefully, and scaling the application to handle high volumes of transactions.
How can I optimize CRUD operations for better performance?
Optimizing CRUD operations for better performance can involve using prepared statements for databases, implementing caching mechanisms, and minimizing database access by bundling operations.
Source code of crud operation in java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.ArrayList;
import java.util.Scanner;
class InventoryManagementSystem extends JFrame {
private ArrayList<String> inventory = new ArrayList<>();
private JTextField itemField;
private JTextArea displayArea;
private final String DATA_FILE = "savaData.txt";
public InventoryManagementSystem() {
loadInventoryFromFile();
createUI();
}
private void createUI() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 400);
setLocationRelativeTo(null);
setLayout(new BorderLayout());
// Create panels
JPanel topPanel = new JPanel();
JPanel centerPanel = new JPanel();
// Create buttons
JButton enterDataButton = new JButton("Enter Data");
JButton showDataButton = new JButton("Show Data");
JButton saveDataButton = new JButton("Save Data");
JButton updateDataButton = new JButton("Update Data");
JButton deleteDataButton = new JButton("Delete Data"); // Delete data button
// Create text field and area
itemField = new JTextField(20);
displayArea = new JTextArea(10, 50);
displayArea.setEditable(false);
// Add components to panels
topPanel.add(enterDataButton);
topPanel.add(showDataButton);
topPanel.add(saveDataButton);
topPanel.add(updateDataButton);
topPanel.add(deleteDataButton); // Add delete button to top panel
centerPanel.add(new JScrollPane(displayArea));
// Add panels to frame
add(topPanel, BorderLayout.NORTH);
add(centerPanel, BorderLayout.CENTER);
// Action listeners
enterDataButton.addActionListener(e -> onEnterData());
showDataButton.addActionListener(e -> onShowData());
saveDataButton.addActionListener(e -> onSaveData());
updateDataButton.addActionListener(e -> onUpdateData());
deleteDataButton.addActionListener(e -> onDeleteData()); // Add action listener for delete button
}
private void onEnterData() {
String item = JOptionPane.showInputDialog(this, "Enter item:");
if (item != null && !item.isEmpty()) {
inventory.add(item);
JOptionPane.showMessageDialog(this, "Item added.");
}
}
private void onShowData() {
displayArea.setText("");
for (String item : inventory) {
displayArea.append(item + "\n");
}
}
private void onSaveData() {
try (FileWriter writer = new FileWriter(DATA_FILE, false)) {
for (String item : inventory) {
writer.write(item + "\n");
}
JOptionPane.showMessageDialog(this, "Data saved to file.");
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "Failed to save data: " + e.getMessage());
}
}
private void loadInventoryFromFile() {
File file = new File(DATA_FILE);
if (file.exists()) {
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextLine()) {
inventory.add(scanner.nextLine());
}
} catch (FileNotFoundException e) {
JOptionPane.showMessageDialog(this, "File not found: " + e.getMessage());
}
}
}
private void onUpdateData() {
String currentData = JOptionPane.showInputDialog(this, "Enter old item:");
if (currentData != null && inventory.contains(currentData)) {
String newData = JOptionPane.showInputDialog(this, "Enter new item for " + currentData + ":");
if (newData != null && !newData.isEmpty()) {
inventory.set(inventory.indexOf(currentData), newData);
JOptionPane.showMessageDialog(this, "Item updated.");
}
} else {
JOptionPane.showMessageDialog(this, "Item not found.");
}
}
private void onDeleteData() {
String itemToDelete = JOptionPane.showInputDialog(this, "Enter item to delete:");
if (itemToDelete != null && inventory.contains(itemToDelete)) {
inventory.remove(itemToDelete);
JOptionPane.showMessageDialog(this, "Item deleted.");
} else {
JOptionPane.showMessageDialog(this, "Item not found.");
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
InventoryManagementSystem frame = new InventoryManagementSystem();
frame.setVisible(true);
});
}
}