Learn how to build an Interactive Server Monitoring Dashboard in Java that tracks real-time server health, performance, and status. This guide covers creating a dynamic dashboard with alerts, server metrics, and an intuitive user interface using Java Swing.
Overview of building an Interactive Server Monitoring Dashboard in Java
The basic functionality of this code is to monitor the health of multiple servers in real-time and display their status in a user-friendly graphical interface. It provides the following core features:
- Server Data Simulation:
- The
Server
class simulates server metrics such as load, CPU usage, memory usage, and availability with random values, which are updated periodically.
- The
- Real-Time Monitoring:
- The
Server Monitor
class updates the server metrics every 5 seconds in the background and checks for critical conditions (e.g., high load or server unavailability). It also triggers alerts when issues are detected.
- The
- Data Display:
- The
Dashboard Controller
class manages the graphical user interface (GUI), displaying server information in a table. It also provides an alerts area that logs any critical issues detected by theServer Monitor
.
- The
- User Interaction:
- The GUI allows users to interact with the system by toggling monitoring on/off and switching between light and dark modes.
- Color-Coded Visualization in java:
- The
TableCellRenderer
class customizes the appearance of the table, using color-coding to represent server health in java and status, making it easy for users to visually identify issues.
- The
Note
You can get the code for Interactive Server Monitoring Dashboard in Java from my GitHub repository
Or you can also Download the code for checking Server Health in java By Clicking on this button
1. Server Class
Purpose:
The Server
class models the properties and behavior of individual servers. Each Server
object stores details such as:
- Name: Identifies the server.
- Load: Indicates server workload on a scale of 0 to 1.
- CPU Usage: A percentage representing the amount of CPU resources used.
- Memory Usage: A percentage representing memory consumption.
- Availability: A boolean flag (true if the server is online, false if offline).
Key Method:
updateStatus()
:- Simulates dynamic server behavior by generating random values for load, CPU usage, memory usage, and availability.
- This gives the illusion of real-time monitoring as metrics change over time.
Example:
For a server named “Server A,” the method may simulate a status like:
- Load: 0.6
- CPU Usage: 55%
- Memory Usage: 70%
- Available: true
2. Server Monitor Class
Purpose:
The Server Monitor
is the control center for server monitoring. It:
- Periodically updates server statuses.
- Triggers alerts for servers in critical conditions.
- Sends data to the
Dashboard Controller
for UI updates.
Components:
startMonitoring()
:- Starts a background
Timer
that refreshes server statuses every 5 seconds. - Calls
updateStatus()
on all servers and checks for alert conditions.
- Starts a background
stopMonitoring()
:- Stops the timer, effectively pausing the monitoring process.
checkForAlerts(Server server)
:- Evaluates server health and raises alerts if:
- The load exceeds 80% (0.8).
- The server is unavailable
- Evaluates server health and raises alerts if:
Interaction:
This class communicates with the Dashboard Controller
to:
- Update the server table dynamically.
- Append new alerts to the alerts area.
3. Dashboard Controller Class
Purpose:
The Dashboard Controller
manages the user interface (UI). It acts as a bridge between the backend logic and the GUI.
Key Responsibilities:
- Server Table:
- Uses a
DefaultTableModel
to display server metrics like name, load, CPU usage, memory usage, and availability. - Dynamically updates rows with the latest server data.
- Uses a
- Alerts Area:
- A
JTextArea
is used to log alerts. - Alerts are timestamped and displayed in a scrollable area for real-time feedback.
- A
- Control Panel:
- Contains buttons for:
- Pause/Resume Monitoring: Starts or stops the monitoring process.
- Dark Mode Toggle: Switches between light and dark themes.
- Contains buttons for:
- Dark Mode Support:
- A
boolean
variable (isDarkMode
) tracks the current theme. - Ensures the UI elements like table cells are rendered with appropriate colors.
- A
- UI Updates:
updateDisplay(Server server)
: Updates the server table with new data.showAlert(String message)
: Adds new alerts to the alerts area.
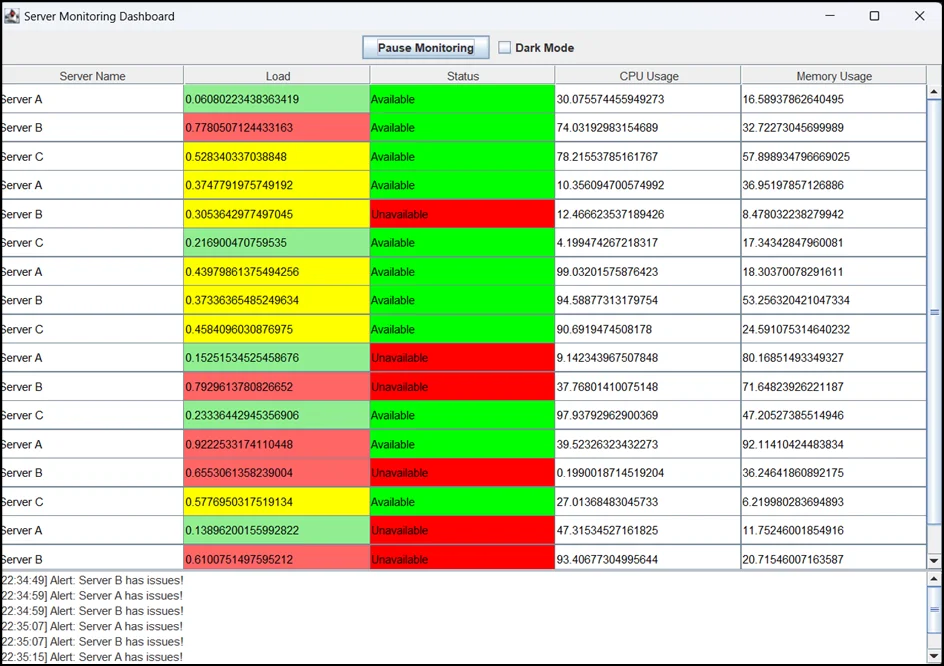
4. TableCellRenderer Class
Purpose:
The TableCellRenderer
customizes the appearance of table cells based on server metrics. It enhances the user experience by:
- Color Coding:
- Load Levels:
- Green for low load (<30%).
- Yellow for medium load (30–60%).
- Red for high load (>60%).
- Availability:
- Green for “Available.”
- Red for “Unavailable.”
- Load Levels:
- Dark Mode Compatibility:
- Adjusts background and text colors for better visibility in dark mode.
Example:
If a server’s load is 85%, its cell will appear red. If the server is unavailable, its status cell will also appear red.
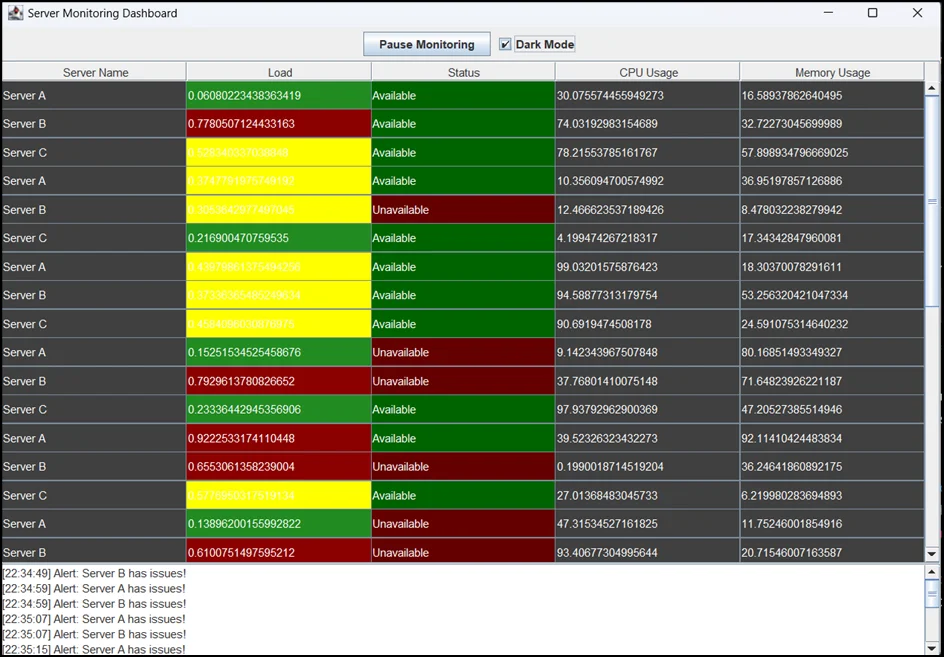
5. Main Class
Purpose:
The Main
class initializes the application and brings all components together. It sets up the JFrame and arranges the GUI elements.
Workflow:
- Create Servers:
- Initializes a list of servers (
Server A
,Server B
,Server C
). - Each server is initialized with:
- Random metrics for load, CPU usage, memory usage, and availability
- Initializes a list of servers (
- Initialize Monitoring:
- Creates a
ServerMonitor
to track server statuses. - Starts monitoring by calling
startMonitoring()
.
- Creates a
- Build the GUI:
- Uses a
JFrame
as the main window. - Adds the following components:
- Table: Displays server metrics in the center.
- Alerts Area: Logs alerts in a scrollable pane at the bottom.
- Control Panel: Buttons and toggles for user interaction at the top.
- Uses a
- Event Handling:
- Configures actions for buttons (e.g., toggling monitoring and dark mode).
6. How Components Interact
Real-Time Monitoring Flow in java:
- The
ServerMonitor
updates each server’s metrics by callingupdateStatus()
. - It checks for critical conditions and raises alerts if necessary.
- The
DashboardController
:- Updates the server table with new data.
- Logs alerts in the alerts area.
- The
TableCellRenderer
ensures the table cells are color-coded based on the new data.
User Interaction:
- Pause/Resume Monitoring:
- Users can pause the monitoring process via the control panel.
- Dark Mode Toggle:
- Switches the UI theme between light and dark.
- Automatically refreshes the table to apply the new theme.
Benefits of Each Component
- Server Class:
- Server Representation: Models a server with key attributes like load, CPU usage, and memory usage, enabling easy tracking and updating of server health metrics.
- Dynamic Updates: Randomized data simulates server activity, providing a realistic test environment for monitoring tools.
- ServerMonitor:
- Real-Time Monitoring: Runs the background process to continuously update server metrics and trigger alerts, ensuring that the system always reflects current server states.
- Alert System: Identifies problematic servers based on predefined conditions (e.g., high load, unavailability), helping users take action quickly.
- Control Over Monitoring: Allows for pausing and resuming the monitoring process, giving flexibility in managing server monitoring.
- DashboardController:
- UI Management: Responsible for updating and displaying real-time server data in the table, managing the alerts area, and controlling user interactions through buttons and toggles.
- User Experience: Simplifies the addition of new server metrics to the display and handles visual updates, such as theme changes and data refreshes.
- Scalability: Centralized control over UI components allows for easy extension (e.g., adding new controls or visual elements).
- TableCellRenderer:
- Custom Table Rendering: Enhances the table’s appearance by providing custom colors and styles based on server status and metrics, improving user experience.
- Theme Support: Adapts to both light and dark mode preferences, ensuring the application is visually appealing under different conditions.
- Clear Data Visualization: Uses color-coding to represent server health (e.g., red for problems, green for healthy), making it easier for users to spot critical issues at a glance.
- Main Class:
- Simplified Application Entry: Acts as the entry point for the program, initializing the necessary components and orchestrating the flow of the entire application.
- Centralized Setup: Creates and links the
DashboardController
,ServerMonitor
, and UI components, ensuring a clean and manageable structure.
How It All Comes Together
The modular design ensures that each component performs a distinct role:
- Backend logic (e.g., server updates and monitoring) is handled by the
Server
andServerMonitor
classes. - UI logic is managed by the
DashboardController
andTableCellRenderer
. - The
Main
class orchestrates these components, resulting in a seamless real-time monitoring dashboard.
Conclusion of an Interactive Server Monitoring Dashboard in Java
This is an Interactive Server Monitoring Dashboard in Java that demonstrates a practical application of object-oriented programming in Java. Each component is designed to work independently, yet integrates seamlessly to deliver a robust and user-friendly solution. By understanding the role of each component, you can not only replicate this project but also extend it to handle real-world scenarios such as actual server metrics and alerts.
Very interesting project, I never knew we could do something like this in java