Learn How to Implement Combat Systems in Java RPGs? This guide takes you through the process of building an interactive story game in Java using Java Swing. You’ll discover how to design turn-based mechanics, manage player and enemy stats, incorporate randomized damage, and create exciting battle sequences. Along the way, we’ll also explore interactive storytelling, inventory management, and adding visual elements to make your RPG game more engaging and immersive.
Key Concepts and Game Mechanics
1. Interactive Storyline
The core of How to Enhance Storytelling in Java RPGs? lies in creating a narrative that adapts to the player’s choices. The game guides players through various scenarios where each decision leads to different outcomes. These decisions are made by interacting with buttons on the graphical user interface (GUI), and the story dynamically evolves based on the player’s selections.
Example:
- Scenario 1: The player wakes up in a dark cave and must choose whether to take a Torch or hide. This choice directly impacts the subsequent events.
- Scenario 2: If the player decides to pick up the Ancient Book, it will increase the player’s intelligence and lead to combat, changing the course of the narrative.
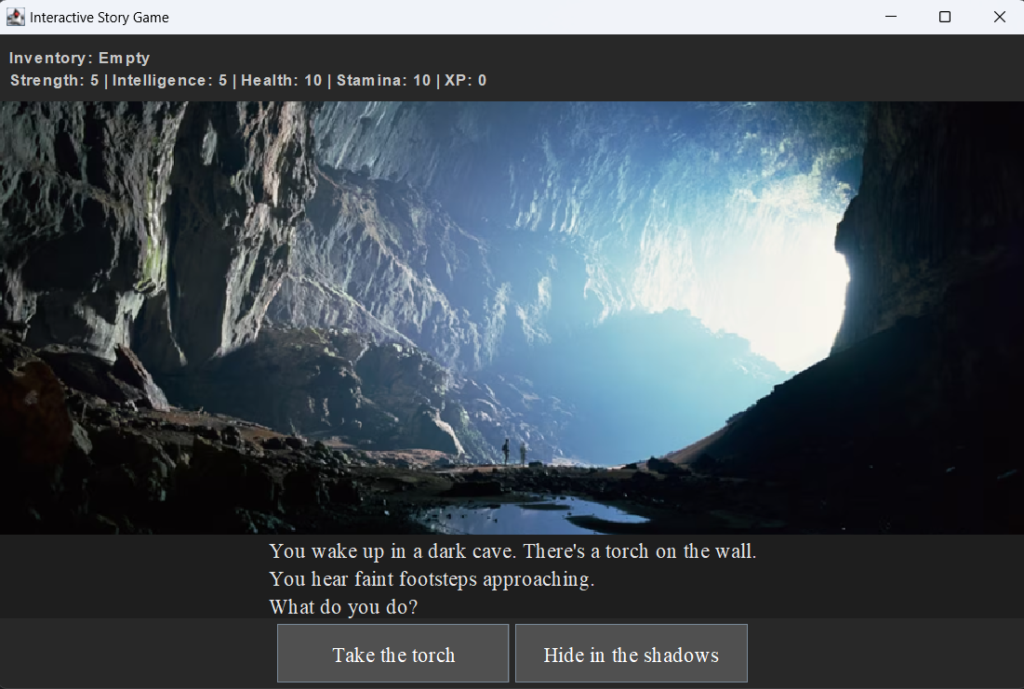
2. Building an Interactive Story Game with Java Swing
The player has a set of attributes like strength, intelligence, health, stamina, and experience points. These attributes can increase or decrease based on the player’s actions and the items they collect. The inventory keeps track of items that the player picks up during the story, and these items can affect the player’s stats.
- Strength affects the player’s combat abilities.
- Intelligence can increase the effectiveness of certain items or abilities.
- Health represents the player’s life, which decreases when taking damage and can be restored using items like potions.
- Stamina determines how much energy the player has to perform actions, such as attacking or fleeing.
- Experience points allow the player to level up, improving their stats.
Example:
- Potion: Adds 5 to the player’s health.
- Stamina Boost: Adds 5 to the player’s stamina.
- Torch: Increases strength.
Each item collected will have a direct impact on one of these stats, creating a personalized and evolving gameplay experience.

3. Combat System
The combat system is turn-based, where both the player and the enemy take turns attacking each other until one of them is defeated. Damage is calculated based on the player’s strength and the enemy’s strength, with a random factor that makes each encounter feel unique.
Example:
- If the player’s strength is 5 and they attack the enemy, the damage could range from 0 to 5 (randomly).
- Similarly, the enemy attacks with a damage range based on their strength.
The player has two main choices during combat:
- Fight: Attack the enemy and deal damage.
- Flee: Escape the encounter, potentially avoiding further damage.
If the player’s health reaches 0, they lose the fight, but if the enemy’s health drops to 0, the player wins and gains experience points.
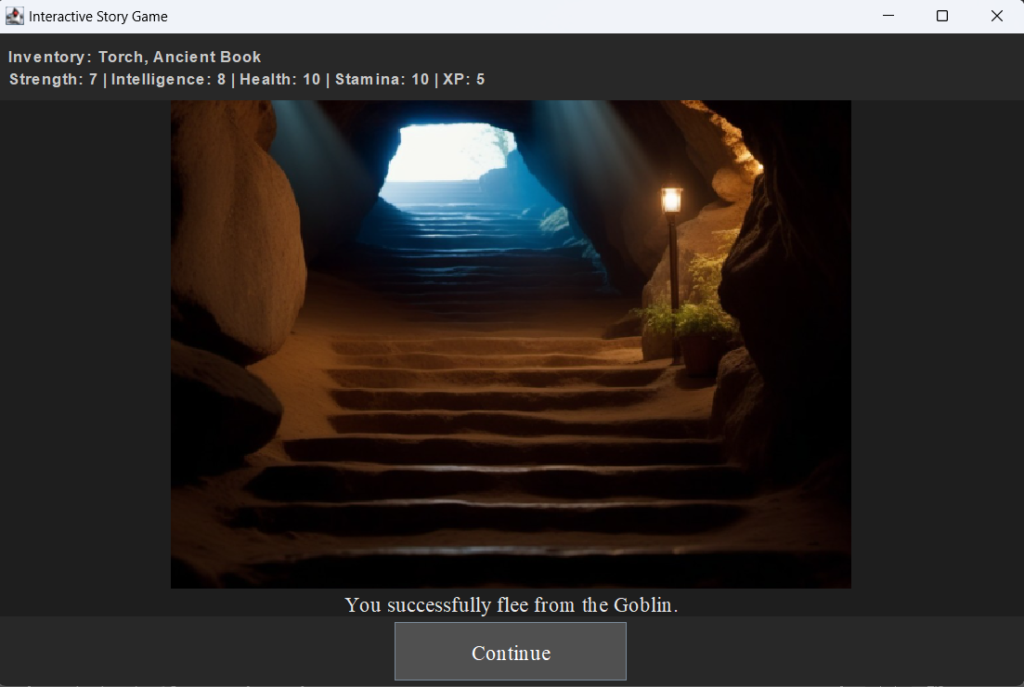
4. Building a Game Framework in Java with GUI
Using Java Swing‘s capabilities, the game integrates images to visually enhance the player’s experience. For example, each decision in the game can be accompanied by an image that depicts the scenario. This adds depth and immersion to the game, helping players feel more connected to the world.
- Images: For each scenario (such as finding a torch or engaging in combat), an image representing the situation is displayed. This could be a dark cave for the starting scenario, or an image of a Goblin during combat.
Note
you can Get the code of “How to Implement Combat Systems in Java RPGs?” from GitHub from my repository
Download the Zip File
Setting Up the Game:
Let’s break down the structure and flow of the game, starting with the user interface and progressing to game logic and interaction.
Step 1: Setting Up the JFrame and UI Elements
The game is built using Swing components like JFrame
, JLabel
, and JButton
. These components form the graphical user interface (GUI), which presents the game’s story, player stats, and inventory, as well as provides buttons for player interactions.
- JFrame: This is the main window where the game runs. It houses all other components and manages the layout.
- JLabel: Used to display text such as the game’s story, player stats, and images.
- JButton: These are clickable buttons that allow the player to make decisions, such as taking the torch, hiding, or fighting an enemy.
Example:
- A
JLabel
is used to display the game’s story, such as:storyLabel.setText("<html>You wake up in a dark cave. There's a torch on the wall.<br>What do you do?</html>");
- The inventory and stats are displayed in a header section, allowing the player to keep track of their progress:
statsLabel.setText("Strength: 5 | Intelligence: 5 | Health: 10 | Stamina: 10"); inventoryLabel.setText("Inventory: Empty");
Step 2: Handling Player Input
When the game starts, the player is prompted to enter their name. Once the name is submitted, the game initializes the player’s stats and starts the first story scenario.
- A
JTextField
collects the player’s name, and aJButton
submits the name.
JTextField nameField = new JTextField(15);
JButton submitButton = createButton("Submit");
The player’s name will personalize the game, and the player can begin making choices immediately.
Step 3: Story and Decision Making
The game proceeds with a branching story. The player makes decisions by clicking buttons like “Take the torch” or “Hide in the shadows”. These decisions trigger different parts of the game and can affect the player’s stats or introduce new challenges (like enemies).
- Each decision has a corresponding event handler:
takeTorch.addActionListener(e -> { player.addItem("Torch"); updateStatsAndInventory(); displayStory2("torch"); });
- Story updates: Based on the player’s choices, the text changes to reflect the next part of the story. For instance:
storyLabel.setText("<html>With the torch in hand, you scare off the footsteps.<br>Do you take the Ancient Book?</html>");
Step 4: Combat Mechanics
After certain events, like picking up the Ancient Book, the player will encounter an enemy. In this game, the enemy is a Goblin with health and strength attributes.
- The combat loop allows the player and enemy to take turns attacking until one is defeated.
- A random factor is introduced into the damage calculation, ensuring no two fights are the same.
player.attack(enemy);
enemy.attack(player);
- If the player wins, they gain experience points and continue the game. If they lose, the game ends.
Step 5: Updating Stats and Inventory
After each significant action, the game updates the player’s stats and inventory. For example, after taking the Torch:
- The player’s strength increases by 2.
- The inventory is updated to reflect the new item:
inventoryLabel.setText("Inventory: " + player.getInventory()); statsLabel.setText(player.getStats());
Conclusion
By the end of this walkthrough, you should have a basic understanding of building an Interactive Story Game with Java Swing. The game combines interactive storytelling, combat, inventory management, and stats tracking to create an engaging user experience. Additionally, the use of images and dynamic story elements enhances the immersion, allowing players to make decisions that impact the outcome of the game.
Source Code of How to Implement Combat Systems in Java RPGs?
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
class Player {
String name;
int strength;
int intelligence;
int health;
int stamina;
int experience;
List<String> inventory;
public Player(String name) {
this.name = name;
this.strength = 5;
this.intelligence = 5;
this.health = 10;
this.stamina = 10;
this.experience = 0;
this.inventory = new ArrayList<>();
}
public void addItem(String item) {
inventory.add(item);
switch (item) {
case "Torch":
strength += 2;
break;
case "Ancient Book":
intelligence += 3;
break;
case "Potion":
health += 5;
break;
case "Stamina Boost":
stamina += 5;
break;
}
}
public void useItem(String item) {
if (inventory.contains(item)) {
switch (item) {
case "Potion":
health += 5;
break;
case "Stamina Boost":
stamina += 5;
break;
}
inventory.remove(item);
}
}
public String getInventory() {
return inventory.isEmpty() ? "Empty" : String.join(", ", inventory);
}
public String getStats() {
return "Strength: " + strength + " | Intelligence: " + intelligence + " | Health: " + health + " | Stamina: " + stamina + " | XP: " + experience;
}
public void gainExperience(int points) {
experience += points;
if (experience >= 10) {
levelUp();
}
}
private void levelUp() {
strength += 1;
intelligence += 1;
health += 2;
stamina += 2;
experience = 0; // Reset XP after leveling up
}
// Attack method added to reduce enemy health
public void attack(Enemy enemy) {
int damage = (int) (Math.random() * strength); // Random damage based on player strength
enemy.health -= damage;
if (enemy.health < 0) enemy.health = 0; // Ensure enemy health doesn't go below zero
}
}
class Enemy {
String name;
int health;
int strength;
public Enemy(String name, int health, int strength) {
this.name = name;
this.health = health;
this.strength = strength;
}
// Enemy can attack player
public void attack(Player player) {
int damage = (int) (Math.random() * strength); // Random damage based on enemy strength
player.health -= damage;
if (player.health < 0) player.health = 0; // Ensure player health doesn't go below zero
}
}
class InteractiveStoryGameWithImages {
private JFrame frame;
private JLabel storyLabel;
private JLabel imageLabel;
private JPanel buttonPanel;
private JLabel inventoryLabel;
private JLabel statsLabel;
private Player player;
private Enemy enemy;
private Random random;
public InteractiveStoryGameWithImages() {
random = new Random();
setupUI();
}
private void setupUI() {
frame = new JFrame("Interactive Story Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(900, 600);
frame.setLayout(new BorderLayout());
frame.getContentPane().setBackground(new Color(30, 30, 30));
// Header Panel
JPanel headerPanel = new JPanel(new GridLayout(2, 1));
headerPanel.setBorder(new EmptyBorder(10, 10, 10, 10));
headerPanel.setBackground(new Color(40, 40, 40));
inventoryLabel = new JLabel("Inventory: Empty");
inventoryLabel.setFont(new Font("SansSerif", Font.BOLD, 14));
inventoryLabel.setForeground(new Color(200, 200, 200));
statsLabel = new JLabel("Stats: Strength: 5 | Intelligence: 5 | Health: 10 | Stamina: 10");
statsLabel.setFont(new Font("SansSerif", Font.BOLD, 14));
statsLabel.setForeground(new Color(200, 200, 200));
headerPanel.add(inventoryLabel);
headerPanel.add(statsLabel);
// Story and Image Panel
JPanel storyImagePanel = new JPanel(new BorderLayout());
storyImagePanel.setBackground(new Color(30, 30, 30));
imageLabel = new JLabel();
imageLabel.setHorizontalAlignment(SwingConstants.CENTER);
storyLabel = new JLabel("<html>Welcome to the Interactive Story Game!<br>Enter your name to begin:</html>");
storyLabel.setFont(new Font("Serif", Font.PLAIN, 18));
storyLabel.setForeground(new Color(230, 230, 230));
storyLabel.setHorizontalAlignment(SwingConstants.CENTER);
storyImagePanel.add(imageLabel, BorderLayout.CENTER);
storyImagePanel.add(storyLabel, BorderLayout.SOUTH);
// Button Panel
buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout());
buttonPanel.setBackground(new Color(40, 40, 40));
frame.add(headerPanel, BorderLayout.NORTH);
frame.add(storyImagePanel, BorderLayout.CENTER);
frame.add(buttonPanel, BorderLayout.SOUTH);
startGame();
frame.setVisible(true);
}
private void startGame() {
buttonPanel.removeAll();
JTextField nameField = new JTextField(15);
JButton submitButton = createButton("Submit");
submitButton.addActionListener(e -> {
String name = nameField.getText().trim();
if (!name.isEmpty()) {
player = new Player(name);
updateStatsAndInventory();
displayStory1();
} else {
storyLabel.setText("<html>Name cannot be empty. Please try again.</html>");
}
});
buttonPanel.add(nameField);
buttonPanel.add(submitButton);
frame.revalidate();
frame.repaint();
}
private void displayStory1() {
storyLabel.setText("<html>You wake up in a dark cave. There's a torch on the wall.<br>You hear faint footsteps approaching.<br>What do you do?</html>");
setImage("pic1.png");
buttonPanel.removeAll();
JButton takeTorch = createButton("Take the torch");
JButton hide = createButton("Hide in the shadows");
takeTorch.addActionListener(e -> {
player.addItem("Torch");
updateStatsAndInventory();
displayStory2("torch");
});
hide.addActionListener(e -> displayStory2("hide"));
buttonPanel.add(takeTorch);
buttonPanel.add(hide);
frame.revalidate();
frame.repaint();
}
private void displayStory2(String decision) {
if (decision.equals("torch")) {
storyLabel.setText("<html>With the torch in hand, you scare off the footsteps.<br>You find an ancient book on a pedestal.<br>Do you take it?</html>");
setImage("pic2.png");
} else {
storyLabel.setText("<html>You hide and the footsteps fade away.<br>You find an ancient book on a pedestal.<br>Do you take it?</html>");
setImage("pic3.png");
}
buttonPanel.removeAll();
JButton takeBook = createButton("Take the book");
JButton leaveBook = createButton("Leave the book");
takeBook.addActionListener(e -> {
player.addItem("Ancient Book");
player.gainExperience(5); // Gain XP for taking the book
updateStatsAndInventory();
startCombat(); // Start combat after taking the book
});
leaveBook.addActionListener(e -> startCombat()); // Start combat if book is left
buttonPanel.add(takeBook);
buttonPanel.add(leaveBook);
frame.revalidate();
frame.repaint();
}
private void startCombat() {
// Creating a random enemy for combat
enemy = new Enemy("Goblin", 20, 3);
storyLabel.setText("<html>A wild " + enemy.name + " appears! Do you want to fight?</html>");
setImage("pic4.png");
buttonPanel.removeAll();
JButton fightButton = createButton("Fight");
fightButton.addActionListener(e -> {
player.attack(enemy);
if (enemy.health <= 0) {
storyLabel.setText("<html>You defeated the " + enemy.name + "!</html>");
player.gainExperience(10);
updateStatsAndInventory();
endCombat();
} else {
enemy.attack(player);
if (player.health <= 0) {
storyLabel.setText("<html>You were defeated by the " + enemy.name + "...</html>");
endCombat();
} else {
startCombat(); // Continue combat if both are still alive
}
}
});
JButton fleeButton = createButton("Flee");
fleeButton.addActionListener(e -> {
storyLabel.setText("<html>You successfully flee from the " + enemy.name + ".</html>");
endCombat();
});
buttonPanel.add(fightButton);
buttonPanel.add(fleeButton);
frame.revalidate();
frame.repaint();
}
private void endCombat() {
buttonPanel.removeAll();
JButton continueButton = createButton("Continue");
continueButton.addActionListener(e -> {
storyLabel.setText("<html>What do you want to do next?</html>");
updateStatsAndInventory();
frame.revalidate();
frame.repaint();
});
buttonPanel.add(continueButton);
frame.revalidate();
frame.repaint();
}
private JButton createButton(String text) {
JButton button = new JButton(text);
button.setFont(new Font("Serif", Font.PLAIN, 18));
button.setBackground(new Color(80, 80, 80));
button.setForeground(Color.WHITE);
button.setFocusPainted(false);
button.setPreferredSize(new Dimension(200, 50));
return button;
}
private void setImage(String imagePath) {
ImageIcon icon = new ImageIcon(imagePath); // Make sure these images exist
imageLabel.setIcon(icon);
}
private void updateStatsAndInventory() {
inventoryLabel.setText("Inventory: " + player.getInventory());
statsLabel.setText(player.getStats());
}
public static void main(String[] args) {
SwingUtilities.invokeLater(InteractiveStoryGameWithImages::new);
}
}