In this post, we will learn how to create a C++ project for Trading Management System that allows users to manage trading accounts. The system will support basic operations such as adding, displaying, modifying, and deleting accounts. The accounts’ data will be stored in a binary file, ensuring persistence across program executions.
Prerequisites
To follow along with this tutorial, you should have a basic understanding of C++ and be familiar with concepts like classes, file I/O, and vectors.
Classes of Trading Management System
The code is divided into two main classes. TradingAccount
and TradingManagementSystem
.
- The
TradingAccount
class represents a single trading account, with attributes such as account ID, name, account type, and balance. - The
TradingManagementSystem
class, on the other hand, represents the entire trading management system, with methods for adding, modifying, and deleting accounts
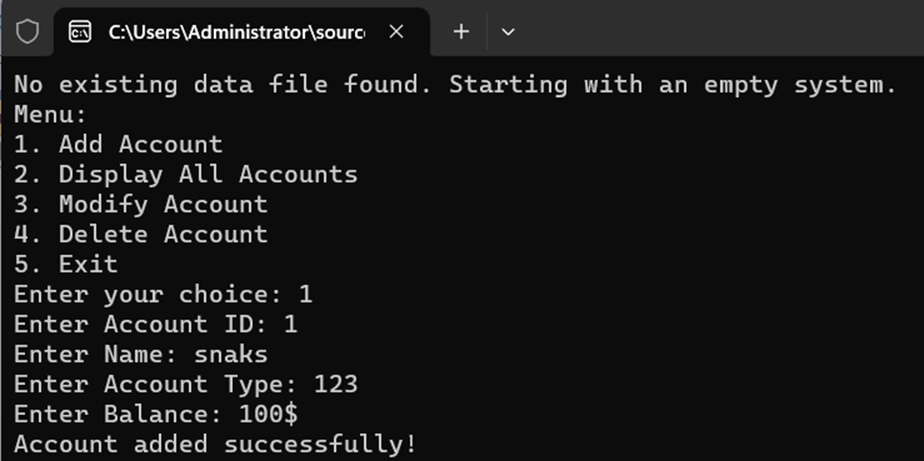
Trading Account Class
The TradingAccount
class is a simple class that represents a single trading account. It has four attributes: accountId
, name
, accountType
, and balance
. The displayAccountDetails()
method is used to display the account details in a formatted way.
- Include Necessary Headers:
iostream
: For input and output operations.iomanip
: For setting the precision of floating-point output.string
: For using thestring
class.
- Class Definition:
- The
TradingAccount
class contains four public members:accountId
,name
,accountType
, andbalance
. - The
displayAccountDetails
method prints the account’s details, with the balance displayed to two decimal places usingfixed
andsetprecision
.
- The
#include <iostream>
#include <iomanip>
#include <string>
using namespace std;
class TradingAccount {
public:
int accountId;
string name;
string accountType; // e.g., "Savings", "Checking"
float balance;
void displayAccountDetails() const {
cout << "Account ID: " << accountId << "\nName: " << name << "\nAccount Type: " << accountType
<< "\nBalance: $" << fixed << setprecision(2) << balance << endl;
}
};
Trading Management System Class
The TradingManagementSystem
class is the heart of the trading management system. It has a vector of TradingAccount
objects, which represents the list of all accounts in the system. The class has several methods:
- Class Definition:
- The
TradingManagementSystem
class contains a private vectoraccounts
to store multipleTradingAccount
objects. - The private member
filename
holds the name of the file used for saving and loading account data.
- The
- Methods:
addAccount
: Prompts the user to enter account details, adds the new account to the vector, and saves the data to the file.displayAllAccounts
: Displays details of all accounts. If no accounts are available, it prints a message indicating this.modifyAccount
: Prompts the user to enter a new name, account type, and balance for a specific account ID and updates the account’s details. If the account is not found, it prints a message.deleteAccount
: Deletes an account with the specified account ID. If the account is not found, it prints a message.loadDataFromFile
: Loads account data from the binary file into the vector. If no file is found, it prints a message.saveDataToFile
: Saves account data from the vector to the binary file.~TradingManagementSystem
: Destructor that ensures data is saved to the file when the object is destroyed.
NOTE
Get this code from GitHub repository
Or download this zip file
Main Function
The main()
function creates an instance of the TradingManagementSystem
class and loads account data from a file using loadDataFromFile()
. It then enters a loo p where the user can interact with the system using a menu-driven interface.
Features and Functionality
The trading management system has the following features and functionality:
- Add new accounts: users can add new accounts to the system, including account ID, name, account type, and balance.
- Modify existing accounts: users can modify existing accounts, including updating the name, account type, and balance.
- Delete accounts: users can delete existing accounts from the system.
- Display all accounts: users can view a list of all accounts in the system.
- Load and save data: the system loads account data from a file and saves it to a file when the user exits.
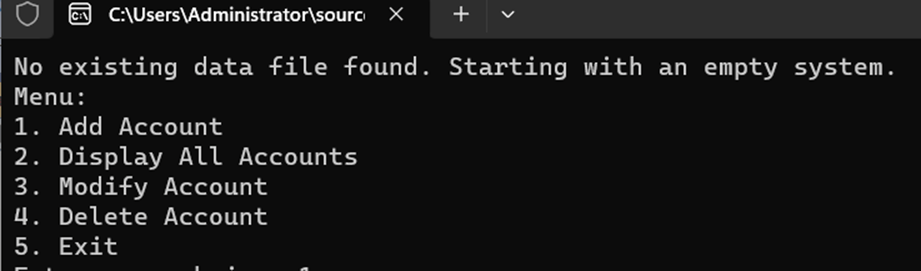
File Input/Output
The system uses binary files to store and load account data. The loadDataFromFile()
method reads the account data from the file and creates a new TradingAccount
object for each account. The saveDataToFile()
method writes the account data from the accounts
vector to the file.
Conclusion
Building a trading management system in C++ can be a complex task, but by breaking it down into smaller components and using object-oriented programming principles, it becomes more manageable. This implementation provides a basic framework for a trading management system, but there are many ways to improve and extend it. Some potential features to add in the future include:
- Implementing security features, such as password protection and encryption
- Adding business logic and rules for trading, such as validating transactions and enforcing account limits
- Creating a user-friendly interface, such as a graphical user interface (GUI)
Source code of C++ project for trading management System
This example of Trading Management System can be extended with additional features such as searching for accounts, performing transactions, or generating reports based on your requirements.
#include <iostream>
#include <iomanip>
#include <vector>
#include <fstream>
#include <string>
using namespace std;
class TradingAccount {
public:
int accountId;
string name;
string accountType; // e.g., "Savings", "Checking"
float balance;
void displayAccountDetails() const {
cout << "Account ID: " << accountId << "\nName: " << name << "\nAccount Type: " << accountType << "\nBalance: $" << fixed << setprecision(2) << balance << endl;
}
};
class TradingManagementSystem {
private:
vector<TradingAccount> accounts;
const string filename = "trading_data.dat";
public:
void addAccount() {
TradingAccount newAccount;
cout << "Enter Account ID: ";
cin >> newAccount.accountId;
cin.ignore(); // Clear newline from buffer
cout << "Enter Name: ";
getline(cin, newAccount.name);
cout << "Enter Account Type: ";
getline(cin, newAccount.accountType);
cout << "Enter Balance: ";
cin >> newAccount.balance;
accounts.push_back(newAccount);
saveDataToFile();
cout << "Account added successfully!" << endl;
}
void displayAllAccounts() const {
if (accounts.empty()) {
cout << "No accounts available in the system." << endl;
return;
}
cout << "Account List:\n";
for (const TradingAccount& account : accounts) {
account.displayAccountDetails();
cout << "------------------------\n";
}
}
void modifyAccount(int accountId) {
for (TradingAccount& account : accounts) {
if (account.accountId == accountId) {
cout << "Modify Account ID " << accountId << ":\n";
cout << "Enter New Name: ";
cin.ignore(); // Clear newline from buffer
getline(cin, account.name);
cout << "Enter New Account Type: ";
getline(cin, account.accountType);
cout << "Enter New Balance: ";
cin >> account.balance;
saveDataToFile();
cout << "Account modified successfully!" << endl;
return;
}
}
cout << "Account with ID " << accountId << " not found." << endl;
}
void deleteAccount(int accountId) {
for (auto it = accounts.begin(); it != accounts.end(); ++it) {
if (it->accountId == accountId) {
accounts.erase(it);
saveDataToFile();
cout << "Account deleted successfully!" << endl;
return;
}
}
cout << "Account with ID " << accountId << " not found." << endl;
}
void loadDataFromFile() {
ifstream inFile(filename, ios::binary);
if (!inFile) {
cout << "No existing data file found. Starting with an empty system." << endl;
return;
}
while (!inFile.eof()) {
TradingAccount loadedAccount;
inFile.read(reinterpret_cast<char*>(&loadedAccount), sizeof(TradingAccount));
if (inFile.eof()) {
break;
}
accounts.push_back(loadedAccount);
}
inFile.close();
}
void saveDataToFile() const {
ofstream outFile(filename, ios::binary);
for (const TradingAccount& account : accounts) {
outFile.write(reinterpret_cast<const char*>(&account), sizeof(TradingAccount));
}
outFile.close();
}
~TradingManagementSystem() {
saveDataToFile();
}
// Add more functionalities like search, transaction, etc., based on your requirements
};
int main() {
TradingManagementSystem tradingSystem;
tradingSystem.loadDataFromFile();
char choice;
do {
cout << "Menu:\n1. Add Account\n2. Display All Accounts\n3. Modify Account\n4. Delete Account\n5. Exit\nEnter your choice: ";
cin >> choice;
switch (choice) {
case '1':
tradingSystem.addAccount();
break;
case '2':
tradingSystem.displayAllAccounts();
break;
case '3': {
int accountId;
cout << "Enter Account ID to modify: ";
cin >> accountId;
tradingSystem.modifyAccount(accountId);
break;
}
case '4': {
int accountId;
cout << "Enter Account ID to delete: ";
cin >> accountId;
tradingSystem.deleteAccount(accountId);
break;
}
case '5':
cout << "Exiting the Trading Management System. Thank you!\n";
break;
default:
cout << "Invalid choice. Please try again.\n";
}
} while (choice != '5');
return 0;
}