In this article, we will create a simple calculator project in Java Swing on IntelliJ idea step-by-step guide. This project serves as an excellent opportunity for Java students, especially those learning GUI programming, to develop a practical and interactive application. By following along with this tutorial, you’ll gain valuable insights into event handling, layout management, and user interface design using Java Swing.
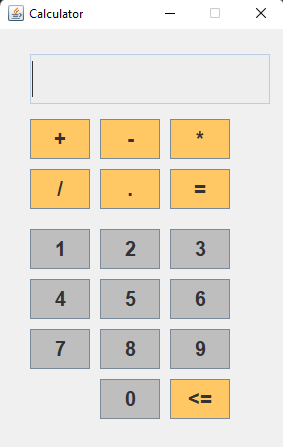
Key Features
First of all, discuss the key points of that Java Swing calculator. This project will give a user-friendly interface, following basic arithmetic operations, decimal point support, error handling and event handling, and Clear and Delete functionalities. Let us discuss them step-by-step.
User-friendly Interface:
The calculator features a clean and intuitive user interface designed with Swing components for seamless interaction.
Basic Arithmetic Operations:
Users can perform addition, subtraction, multiplication, and division operations with ease.
Decimal Point Support:
The calculator supports decimal numbers, allowing for precise calculations.
Error Handling:
The application gracefully handles division by zero and displays an error message to the user.
Clear and Delete Functionality:
Users can clear the input field or delete the last character with the click of a button.
Source code
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class SwingCalculator extends JFrame implements ActionListener {
private JTextField textField;
private JButton[] numberButtons;
private JButton[] functionButtons;
private JPanel panel;
private double num1 = 0, num2 = 0, result = 0;
private char operator;
SwingCalculator() {
setTitle("Calculator");
setSize(300, 500);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
panel = new JPanel();
panel.setLayout(null);
panel.setBackground(new Color(240, 240, 240));
textField = new JTextField();
textField.setBounds(30, 25, 240, 50);
textField.setFont(new Font("Arial", Font.BOLD, 30));
textField.setEditable(false);
panel.add(textField);
numberButtons = new JButton[10];
for (int i = 0; i < 10; i++) {
numberButtons[i] = new JButton(String.valueOf(i));
numberButtons[i].setFont(new Font("Arial", Font.BOLD, 20));
numberButtons[i].setBackground(new Color(190, 190, 190));
numberButtons[i].addActionListener(this);
}
functionButtons = new JButton[8];
functionButtons[0] = new JButton("+");
functionButtons[1] = new JButton("-");
functionButtons[2] = new JButton("*");
functionButtons[3] = new JButton("/");
functionButtons[4] = new JButton(".");
functionButtons[5] = new JButton("=");
functionButtons[6] = new JButton("<=");
functionButtons[7] = new JButton("Clear");
for (JButton button : functionButtons) {
button.setFont(new Font("Arial", Font.BOLD, 20));
button.setBackground(new Color(255, 200, 100));
button.addActionListener(this);
}
int buttonX = 30;
int buttonY = 90;
for (int i = 0; i < 7; i++) {
functionButtons[i].setBounds(buttonX, buttonY, 60, 40);
panel.add(functionButtons[i]);
buttonX += 70;
if (buttonX >= 190) {
buttonX = 30;
buttonY += 50;
}
}
int numberX = 30;
int numberY = 200;
for (int i = 1; i <= 9; i++) {
if (i == 4 || i == 7) {
numberX = 30;
numberY += 50;
}
numberButtons[i].setBounds(numberX, numberY, 60, 40);
panel.add(numberButtons[i]);
numberX += 70;
}
numberButtons[0].setBounds(100, 350, 60, 40);
panel.add(numberButtons[0]);
functionButtons[6].setBounds(170, 350, 60, 40); // Adjusted size of "Delete" button
panel.add(functionButtons[6]);
getContentPane().add(panel);
setVisible(true);
}
public static void main(String[] args) {
new SwingCalculator();
}
@Override
public void actionPerformed(ActionEvent e) {
for (int i = 0; i < 10; i++) {
if (e.getSource() == numberButtons[i]) {
textField.setText(textField.getText().concat(String.valueOf(i)));
}
}
if (e.getSource() == functionButtons[4]) {
textField.setText(textField.getText().concat("."));
}
if (e.getSource() == functionButtons[0]) {
num1 = Double.parseDouble(textField.getText());
operator = '+';
textField.setText("");
}
if (e.getSource() == functionButtons[1]) {
num1 = Double.parseDouble(textField.getText());
operator = '-';
textField.setText("");
}
if (e.getSource() == functionButtons[2]) {
num1 = Double.parseDouble(textField.getText());
operator = '*';
textField.setText("");
}
if (e.getSource() == functionButtons[3]) {
num1 = Double.parseDouble(textField.getText());
operator = '/';
textField.setText("");
}
if (e.getSource() == functionButtons[5]) {
num2 = Double.parseDouble(textField.getText());
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0)
result = num1 / num2;
else
textField.setText("Error");
break;
}
textField.setText(String.valueOf(result));
num1 = result;
}
if (e.getSource() == functionButtons[7]) {
textField.setText("");
}
if (e.getSource() == functionButtons[6]) {
String string = textField.getText();
textField.setText("");
for (int i = 0; i < string.length() - 1; i++) {
textField.setText(textField.getText() + string.charAt(i));
}
}
}
}
GitHub Repository
You can also get that full code from our GitHub repository and also for many other interesting projects
Step-by-step implementation of calculator project using Java Swing
Now, check out the step-by-step implementation of that Java Swing Calculator.
Setting Up the GUI:
We begin by creating a JFrame window and adding Swing components such as JTextFields for input and JButtons for numbers and operations.
setTitle("Calculator");
setSize(380, 550);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
panel = new JPanel();
panel.setLayout(null);
panel.setBackground(Color.LIGHT_GRAY);
textField = new JTextField();
textField.setBounds(30, 25, 310, 50);
textField.setFont(new Font("Arial", Font.BOLD, 30));
textField.setEditable(false);
panel.add(textField);
getContentPane().add(panel);
setVisible(true);
Event Handling:
We implement the ActionListener interface to handle button clicks and perform corresponding actions based on user input.
// Implementing ActionListener interface
public class SwingCalculator extends JFrame implements ActionListener {
// ActionListener interface is implemented in this class
}
Number Input:
When a number button is clicked, its value is appended to the input field.
Decimal Point and Arithmetic Operations:
Users can input decimal numbers and select arithmetic operations (+, -, *, /) to perform calculations.
Error Handling:
Division by zero is detected, and an error message is displayed to the user.
Clear and Delete Functionality:
Buttons for clearing the input field and deleting the last character are implemented.
FAQ
1. What is SwingCalculator?
SwingCalculator is a simple calculator application built using Java Swing, which provides a graphical user interface for performing basic arithmetic operations.
2. How do I use SwingCalculator?
Simply, run the SwingCalculator application, and you’ll see a window displaying a numeric keypad, along with buttons for arithmetic operations (+, -, *, /), decimal point, delete (Del), and clear (Clear). You can input numbers and perform operations using the mouse or keyboard.
3. What happens if I divide by zero?
If you attempt to divide by zero, an error message (“Error”) will be displayed in the input field, indicating that the operation is invalid.