An array is a fundamental data structure in C++ that allows you to store a collection of elements of the same data type.
Table of Contents
ToggleArrays have a fixed size, meaning the number of elements they can hold is determined at the time of declaration. The memory storage for array elements is contiguous, and can be accessed through an index. After the importance of arrays we will discus about the basic operations in arrays.
To understand the basic concept of arrays we have a following terms:
Element: every entry of data stored in an array called element
Index: To locate and reference individual elements, an array relies on numerical indices.
Note: If you want to clone that code as it is then here is the link for our GitHub Repository.
Syntax of Arrays
data_type array_name[array_size];
For example: an array of integer can be declared as follows:
Compiler will allocate a contiguous memory lock of size =6*sizeof(int);
Declaration and Initialization
You can declare and initialize arrays using various methods, such as specifying the size and elements explicitly or dynamically allocating memory for arrays.
int myArray[5] = {1, 2, 3, 4, 5}; // Static initialization
int* dynamicArray = new int[10]; // Dynamic allocation
Initialization of arrays

Types of Arrays
There are three types of arrays:
- One Dimensional
- Multi-Dimensional

One Dimensional
One-dimensional arrays require only one subscript to specify elements in an array.
In a one-dimensional space, you have only one independent variable. It could be represented as a straight line where points are located based on a single parameter. Examples include time, temperature along a rod, or distance along a road.

Multi-dimensional
In multi-dimensional arrays, you need to use multiple subscripts to identify specific elements.
In multi-dimensional spaces, you have more than one independent variable. Each dimension represents a different parameter, and points are located based on combinations of these parameters.
Examples
2D spaces like a plane (with two independent variables).It is organized in the form of a matrix which can be represented as a collection of rows and columns.
3D spaces like our physical world (with three independent variables), and even higher-dimensional spaces used in mathematics and physics. It is a collection of 2D Arrays which consists of three subscripts:
Block size, row size and column size

Importance of Arrays
- Memory Efficiency: Arrays allocate memory in a contiguous block, which can be more memory-efficient than using separate variables for each data item. This is particularly important when dealing with large data items.
- Index-Based Access: Elements in an array are accessed using their index (position), which is typically an integer. This allows for efficient and direct access to any element in the array.
- Data Organization: Arrays help in organizing and structuring data logically
- Flexibility: Arrays can be used to implement other complex data structures like stacks, queues, and matrices. Many data structures and algorithms build upon the foundation of arrays.
- Reduced Code Repetition: Arrays enable you to manage multiple data items using a single variable name. This reduces code duplication and makes programs more concise and maintainable.
Representation of Array
The elements are stored one after the other, and you can access them using an index.
Additionally, multi-dimensional arrays, such as matrices or tables, are represented as arrays of arrays or with more complex memory layouts to accommodate their structure.
Example
In this example, we have an array of integers with five elements.
The elements are stored one after the other in memory, and each element occupies the same amount of space. The index allows you to access individual elements by specifying their position within the array.
Memory Layout:
+—+—+—+—+—+
| 3 | 7 | 1 | 4 | 9 |
+—+—+—+—+—+
Array Elements:
Index 0: 3
Index 1: 7
Index 2: 1
Index 3: 4
Index 4: 9
Basic Operations in Arrays
Traversal
You can traverse an array using loops like for or while to perform operations on each element.
for (int i = 0; i < 5; i++) {
// Process each element in myArray
}
Insertion
Insertion involves placing one or more elements into an array.
Insertion of an element can be done:
- At the beginning
- At the end
- At any given index of an array.

Accessing Elements
Elements in an array can be accessed using square brackets and an index.
syntax
int element = myArray[2]; // Accessing the third element (index 2)
Modification
You can modify elements in an array by assigning new values to them.
syntax
myArray[3] = 42; // Modifying the fourth element
Searching
Searching is the process of finding a given value in a list of values.
It decides whether the searching key is present in an array or not . It is an algorithmic process of finding a particular item in a collection of data.
Example
int target = 3;
for (int i = 0; i < 5; i++) {
if (myArray[i] == target) {
// Element found
break;
}
}

Appending and Deleting
In C++, arrays have fixed sizes, so adding or removing elements typically involves creating a new array with the desired size and copying elements.
You can sort the elements of an array in ascending or descending order using algorithms like bubble sort, quicksort, or the C++ standard library’s std::sort function.
By default, sorting process is done in the ascending order.
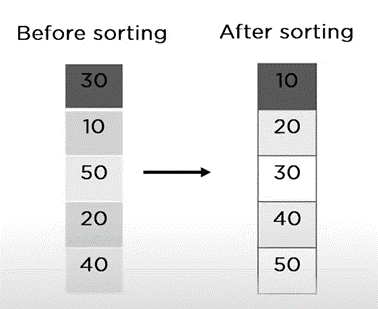
Object-Oriented Approach
In an OOP context, you can create classes to encapsulate array-related functionality, making your code more organized and reusable. For example, you could create a class that represents a dynamic array with methods for adding, removing, and manipulating elements.