In this blog, we will discuss about C++ program to print pyramid and pattern made in the form of stars, numbers, and alphabets by taking input from users in time with full explanations. To understand the full program for printing a pyramid, you must need to have basic knowledge of loops and if-else statements.
Full Star (*) Pyramid
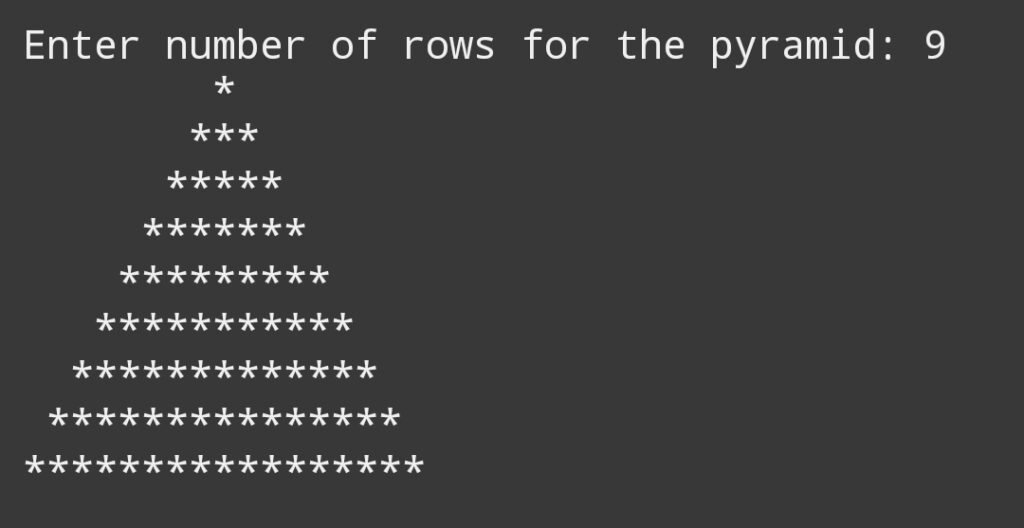
Code for printing pyramid of stars (*)
#include <iostream>
using namespace std;
int main() {
int numRows;
cout << "Enter the number of rows for the pyramid: ";
cin >> numRows;
for (int i = 1; i <= numRows; ++i) {
// Print spaces
for (int j = 1; j <= numRows - i; ++j) {
cout << " ";
}
// Print asterisks
for (int k = 1; k <= 2 * i - 1; ++k) {
cout << "*";
}
// Move to the next line
cout << endl;
}
return 0;
}
Code Explanation
This C++ program prints a star pyramid pattern based on the number of rows specified by the user. It begins by asking the user to input the number of rows for the pyramid. Then, it uses nested loops to construct each row of the pyramid. The outer loop iterates through each row, and within it, there are two inner loops. The first inner loop handles the spaces before the asterisks, ensuring proper alignment, and the second inner loop prints the asterisks for that row. The number of asterisks in each row is determined by the formula 2 * i – 1, where ‘i’ represents the current row number. After completing a row, the program moves to the next line, and this process repeats until the specified number of rows is reached, resulting in a pyramid pattern.
Full Pyramid of Numbers
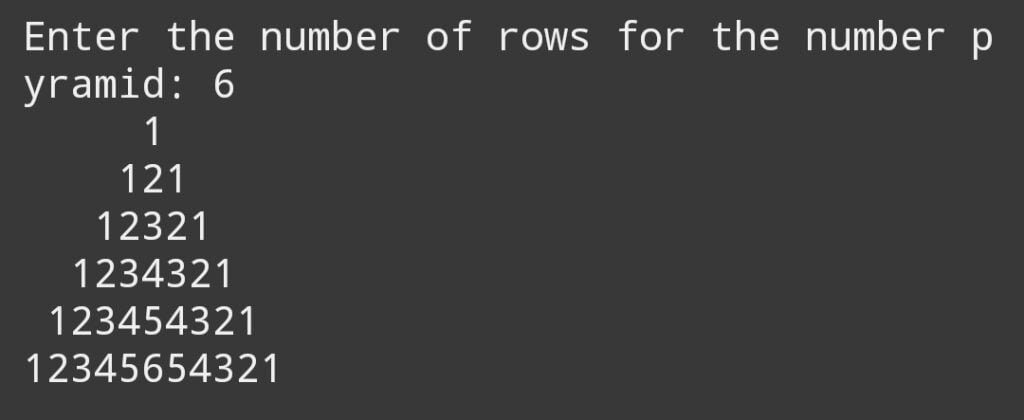
Code for Program to print pyramid and pattern of Numbers
#include <iostream>
using namespace std;
int main() {
int numRows;
cout << "Enter the number of rows for the number pyramid: ";
cin >> numRows;
for (int i = 1; i <= numRows; ++i) {
// Print spaces
for (int j = 1; j <= numRows - i; ++j) {
cout << " ";
}
// Print increasing numbers
for (int k = 1; k <= i; ++k) {
cout << k;
}
// Print decreasing numbers
for (int l = i - 1; l >= 1; --l) {
cout << l;
}
// Move to the next line
cout << endl;
}
return 0;
}
Code Explanation
Program to print pyramid and pattern of numbers based on the user’s input for the number of rows. It starts by prompting the user to enter the desired number of rows for the pyramid. Using nested loops, the program constructs each row of the pyramid. The first inner loop handles printing spaces to ensure proper alignment, and the second inner loop prints increasing numbers up to the current row number. After that, a third inner loop prints decreasing numbers, creating a symmetric pattern. The program repeats this process for each row, and the result is a number pyramid where each row displays a sequence of increasing and then decreasing numbers, centered and aligned properly.
Full Pyramid of Alphabets
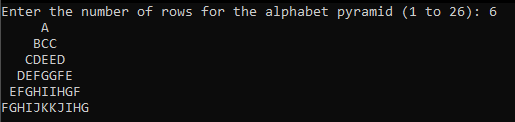
Code for Program to print pyramid of Alphabets
#include <iostream>
using namespace std;
int main() {
int numRows;
cout << "Enter the number of rows for the alphabet pyramid (1 to 26): ";
cin >> numRows;
if (numRows < 1 || numRows > 26) {
cout << "Invalid input. Please enter a number between 1 and 26." << endl;
return 1; // Exit with an error code
}
char currentChar = 'A';
for (int i = 1; i <= numRows; ++i) {
// Print spaces
for (int j = 1; j <= numRows - i; ++j) {
cout << " ";
}
// Print increasing alphabets
for (int k = 1; k <= i; ++k) {
cout << currentChar;
++currentChar;
}
// Print decreasing alphabets
for (int l = i - 1; l >= 1; --l) {
--currentChar;
cout << currentChar;
}
// Move to the next line
cout << endl;
}
return 0;
}
Code Explanation
This C++ program generates an alphabet pyramid pattern based on the user’s input for the number of rows. It starts by prompting the user to enter the desired number of rows for the pyramid, ensuring that the input is within the range of 1 to 26 (since there are 26 letters in the alphabet). Then, using nested loops, the program constructs each row of the pyramid. The first inner loop handles printing spaces to ensure proper alignment, and the second inner loop prints increasing alphabets, starting from ‘A’ and incrementing the character in each iteration. After reaching the maximum character for the row, a third inner loop prints decreasing alphabets, creating a symmetric pattern. The program repeats this process for each row, incrementing and decrementing the character appropriately until the desired number of rows is reached. The result is an alphabet pyramid where each row displays a sequence of increasing and then decreasing alphabets, centered and aligned properly.
Half Pyramid of Numbers (Right-Aligned)
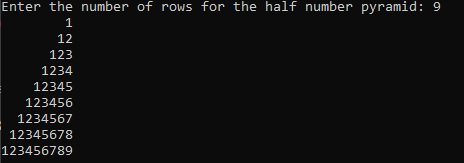
Code Half Pyramid of Numbers
#include <iostream>
using namespace std;
int main() {
int numRows;
cout << "Enter the number of rows for the half number pyramid: ";
cin >> numRows;
for (int i = 1; i <= numRows; ++i) {
// Print spaces
for (int j = 1; j <= numRows - i; ++j) {
cout << " ";
}
// Print increasing numbers
for (int k = 1; k <= i; ++k) {
cout << k;
}
// Move to the next line
cout << endl;
}
return 0;
}
Code Explanation
This C++ program generates a half pyramid pattern where each row contains an increasing number of asterisks, right-aligned. It prompts the user to enter the desired number of rows for the pyramid and constructs each row using nested loops. The outer loop controls the number of rows, while the inner loops handle printing spaces for alignment and asterisks for the pattern. After completing each row, the program moves to the next line. The result is a right-aligned half-pyramid pattern of asterisks.
Half Pyramid of Alphabets
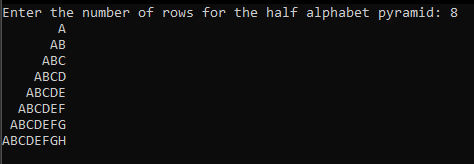
Code of Half Pyramid of Alphabets
#include <iostream>
using namespace std;
int main() {
int numRows;
cout << "Enter the number of rows for the half alphabet pyramid: ";
cin >> numRows;
char currentChar = 'A';
for (int i = 1; i <= numRows; ++i) {
// Print spaces
for (int j = 1; j <= numRows - i; ++j) {
cout << " ";
}
// Print alphabets
for (int k = 1; k <= i; ++k) {
cout << currentChar;
++currentChar;
}
// Move to the next line
cout << endl;
// Reset currentChar for next row
currentChar = 'A';
}
return 0;
}
Code Explanation
This C++ program generates a half pyramid pattern where each row contains an increasing sequence of alphabets (starting from ‘A’) and is right-aligned. It prompts the user to enter the desired number of rows for the pyramid and constructs each row using nested loops. The outer loop controls the number of rows, while the inner loops handle printing spaces for alignment and alphabets for the pattern. After completing each row, the program moves to the next line and resets the character to ‘A’ for the next row. The result is a right-aligned half-pyramid pattern of alphabets.
Half Pyramid of Stars (*)

Code of Half Pyramid of Stars
#include <iostream>
using namespace std;
int main() {
int numRows;
cout << "Enter the number of rows for the half star pyramid: ";
cin >> numRows;
for (int i = 1; i <= numRows; ++i) {
// Print asterisks
for (int k = 1; k <= i; ++k) {
cout << "* ";
}
// Move to the next line
cout << endl;
}
return 0;
}
Code Explanation
This C++ program generates a half-pyramid pattern where each row contains an increasing sequence of asterisks and is right-aligned. It prompts the user to enter the desired number of rows for the pyramid and constructs each row using nested loops. The outer loop controls the number of rows, while the inner loops handle printing spaces for alignment and asterisks for the pattern. After completing each row, the program moves to the next line. The result is a right-aligned half-pyramid pattern of asterisks.
Inverted Pyramid
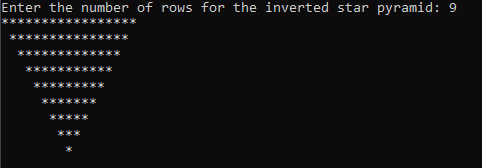
Code of Inverted pyramid of Stars
#include <iostream>
using namespace std;
int main() {
int numRows;
cout << "Enter the number of rows for the inverted star pyramid: ";
cin >> numRows;
for (int i = numRows; i >= 1; --i) {
// Print spaces
for (int j = 0; j < numRows - i; ++j) {
cout << " ";
}
// Print asterisks
for (int k = 1; k <= 2 * i - 1; ++k) {
cout << "*";
}
// Move to the next line
cout << endl;
}
return 0;
}
Code Explanation
This C++ program generates an inverted pyramid pattern where each row contains a decreasing number of asterisks. It prompts the user to enter the desired number of rows for the pyramid and constructs each row using nested loops. The outer loop controls the number of rows, while the inner loops handle printing spaces for alignment and asterisks for the pattern. After completing each row, the program moves to the next line. The result is an inverted pyramid pattern of asterisks.
GitHub
Visit our GitHub repository to find out more interesting and valuable projects with source code for Free.
Comparison
Pyramid Type | Loop Structure | Content Printed | Pattern Symmetry |
---|---|---|---|
Full Star Pyramid | Nested loops: Rows, Spaces, Asterisks | Asterisks (*) | Symmetric |
Half Star Pyramid | Nested loops: Rows, Spaces, Asterisks | Asterisks (*) | Non-symmetric |
Inverted Star Pyramid | Nested loops: Rows, Spaces, Asterisks | Asterisks (*) | Symmetric |
Full Number Pyramid | Nested loops: Rows, Spaces, Numbers | Numbers (1, 2, 3, …) | Symmetric |
Half Number Pyramid | Nested loops: Rows, Spaces, Numbers | Numbers (1, 2, 3, …) | Non-symmetric |
Inverted Number Pyramid | Nested loops: Rows, Spaces, Numbers | Numbers (1, 2, 3, …) | Symmetric |
Full Alphabet Pyramid | Nested loops: Rows, Spaces, Alphabets | Alphabets (A, B, C, …) | Symmetric |
Half Alphabet Pyramid | Nested loops: Rows, Spaces, Alphabets | Alphabets (A, B, C, …) | Non-symmetric |
Inverted Alphabet Pyramid | Nested loops: Rows, Spaces, Alphabets | Alphabets (A, B, C, …) | Symmetric |
FAQ
1: How is a full star pyramid created?
It is created using nested loops in programming, with one loop controlling the number of rows and two inner loops handling spaces and asterisks printing.
2: How is a half-star pyramid different from a full-star pyramid?
Unlike full-star pyramids, half-star pyramids are not symmetric and are aligned to the left.
3: What is a full alphabet pyramid?
A full alphabet pyramid is a symmetrical pattern formed by alphabets arranged in rows, with each row having an increasing sequence of alphabets.