Exception handling in Java is a way to deal with unexpected or error situations that may occur during the execution of a program. It’s like having a safety net to catch and manage problems instead of letting them crash your program.
What is Exception in Programming?
In programming, an exception is an event or occurrence during the execution of a program that disrupts the normal flow of instructions. Exceptions are typically caused by errors or unexpected conditions that arise while a program is running. When an exceptional situation occurs, the normal execution of the program is interrupted, and the control is transferred to a special code segment known as an exception handler.
What are the types of exceptions in Java?
In Java, exceptions are categorized into two main types, Checked exceptions and Unchecked exceptions. Additionally, there is a special type of exception called an error. All these exceptions and errors are under Throwable Class. Let’s explore each of these:
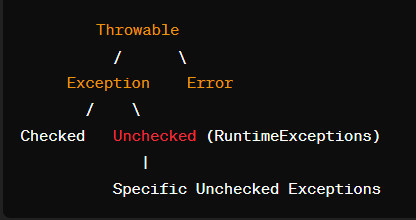
1. Checked Exceptions:
Checked exceptions are exceptions that the Java compiler requires you to catch or declare in the method signature using the throws
clause. These exceptions are typically related to conditions that might occur at runtime, but the compiler checks that they are properly handled.
Examples of Checked Exceptions:
IOException
: Signals that an I/O exception has occurred.SQLException
: Represents an exception that provides information on database access errors.ClassNotFoundException
: Thrown when an application tries to load a class through its string name but no definition for the class with the specified name can be found.
try {
// code that might throw checked exceptions
} catch (IOException e) {
// handle IOException
} catch (SQLException e) {
// handle SQLException
}
2. Unchecked Exceptions (RuntimeExceptions):
Unchecked exceptions, also known as runtime exceptions, are exceptions that the compiler does not require you to catch or declare. They often result from programming errors and represent conditions that occur beyond the control of the programmer.
Examples of Unchecked Exceptions:
NullPointerException
: Thrown when an application attempts to use an object reference that has anull
value.ArrayIndexOutOfBoundsException
: Thrown to indicate that an array has been accessed with an illegal index.ArithmeticException
: Thrown when an exceptional arithmetic condition has occurred.
// Unchecked exceptions are not required to be caught or declared
try {
// code that might throw unchecked exceptions
} catch (NullPointerException e) {
// handle NullPointerException
} catch (ArrayIndexOutOfBoundsException e) {
// handle ArrayIndexOutOfBoundsException
}
3. Errors:
Errors are exceptional conditions that are external to the application, and the application usually cannot anticipate or recover from them. They often indicate a serious problem that should not be caught or handled by the application.
Examples of Errors:
OutOfMemoryError
: Thrown when the Java Virtual Machine (JVM) cannot allocate an object because it is out of memory.StackOverflowError
: Thrown when the execution stack overflows because it becomes too deep.
// Errors are usually not caught or handled by the application
try {
// code that might cause errors
} catch (OutOfMemoryError e) {
// handle OutOfMemoryError (not recommended)
}
Checked VS. Unchecked Exceptions
Feature | Checked Exceptions | Unchecked Exceptions (RuntimeExceptions) |
---|---|---|
Handling Requirement | Must be either caught using a catch block or declared | Optional to catch or declare; the compiler doesn’t force it |
Declared with throws | Required in the method signature if not caught | Not required in the method signature |
Compile-Time Checking | Checked at compile time | Not checked at compile time |
Examples | – IOException <br/> – SQLException | – NullPointerException <br/> – ArrayIndexOutOfBoundsException <br/> – ArithmeticException |
Common Causes | Typically associated with external factors like I/O, database operations, etc. | Often linked to programming errors, like null references or incorrect array indexing |
Derived from Exception | Yes | Yes |
Derived from RuntimeException | No | Yes |
Exception
class. However, unchecked exceptions further extend from RuntimeException
.What is Exception Handling?
Exception handling in Object-Oriented Programming (OOP) is a mechanism that allows programmers to manage and respond to unexpected or exceptional situations during the execution of a program. Exceptions are events that occur during the runtime and disrupt the normal flow of the program. In OOP languages like Java, C++, and Python, exception handling provides a structured way to handle errors or exceptional cases gracefully.
How Exception Handling in Java Works?
In Java, a widely used object-oriented programming language, exception handling is implemented through a combination of special keywords and constructs. The try
block encapsulates the code that might give rise to exceptions, and the catch
block(s) provide a structured way to handle specific types of exceptions, offering developers the flexibility to tailor their responses based on the nature of the error. The throw
keyword allows for the explicit generation of exceptions, enabling developers to communicate exceptional conditions programmatically. Additionally, the optional finally
block provides a space for executing cleanup code, ensuring that certain operations are performed regardless of whether an exception occurs or not.
1. Try Block:
The code that might throw an exception is enclosed in the try
block. This is the risky part of the code where an unexpected situation could occur.
The “Try block” is a fundamental construct in exception handling within programming languages, including Java. It is a block of code that is enclosed by the try
keyword and curly braces ({}
). The primary purpose of the try
block is to encompass a section of code where there is a possibility of an exception being thrown.
try {
// code that might cause an exception
}
2. Exception Occurs:
If an exceptional situation occurs inside the try
block (for example, dividing by zero or accessing an array index out of bounds), an exception object is created. The Throw
keyword is used to explicitly throw an exception. Alternatively, certain operations (like dividing by zero) automatically throw predefined exceptions.
throw new SomeException("This is an exception message");
3. Catch Block:
If an exception occurs, the control is transferred to the appropriate catch
block. The catch
block specifies the type of exception it can handle. Inside the catch
block, you write code to handle the exception. This could include logging the error, displaying a user-friendly message, or taking corrective action. You can have multiple catch
blocks to handle different types of exceptions. The first7. Finally Block (Optional): block that matches the exception type gets executed.
catch (ExceptionType e) {
// code to handle the exception
}
4. Finally Block (Optional):
The finally
block, if present, contains code that always runs, whether an exception occurred or not. It’s often used for cleanup operations. After the catch
block (or finally
block) is executed, the program continues with the code that follows the try-catch
structure.
try {
int result = 10 / 0; // Trying to divide by zero (ArithmeticException)
} catch (ArithmeticException e) {
// Handle the exception
System.out.println("Oops! Something went wrong: " + e.getMessage());
} finally {
// Cleanup code (optional)
}
Related Articles
System Defined Exception Handling
System-defined exceptions, also known as built-in or standard exceptions, are predefined error conditions provided by the programming language or the runtime environment. These exceptions are part of the standard libraries or frameworks and cover common error scenarios. In Java, for instance, system-defined exceptions are part of the java.lang
package.
Java programming handles exceptions and other problems in a strong and reliable manner. These exceptions, which are used to handle unforeseen circumstances and errors during program execution, include SQLException, ClassNotFoundException, IllegalStateException, IllegalArgumentException, and NullPointerException. By preventing SQL syntax problems, dynamic class loading, and other errors, these exceptions contribute to the smooth and effective operation of programs.
1. SQLException:
SQLException is a checked exception that indicates a problem with a database operation. It is often associated with issues such as database connection problems, SQL syntax errors, or violations of integrity constraints.
try {
// Database operations that might cause SQLException
// ...
} catch (SQLException e) {
// Handle SQLException (e.g., log the error, notify the user)
System.out.println("SQLException: " + e.getMessage());
}
2. ClassNotFoundException:
ClassNotFoundException is a checked exception that occurs when trying to load a class at runtime using Class.forName()
but the specified class is not found in the classpath.
try {
// Attempting to load a class that may not exist
Class.forName("com.example.MyClass");
} catch (ClassNotFoundException e) {
// Handle ClassNotFoundException (e.g., log the error, load a default class)
System.out.println("ClassNotFoundException: " + e.getMessage());
}
3. IllegalStateException:
IllegalStateException is an unchecked exception that signals that a method has been invoked at an illegal or inappropriate time or state.
public class StateExample {
private boolean isInitialized = false;
public void initialize() {
if (isInitialized) {
throw new IllegalStateException("Already initialized");
}
// Initialization logic
isInitialized = true;
}
}
4. IllegalArgumentException:
IllegalArgumentException is an unchecked exception that is thrown when a method receives an argument of an inappropriate type or value.
public class ArgumentExample {
public void processNumber(int num) {
if (num < 0) {
throw new IllegalArgumentException("Number must be non-negative");
}
// Process the number
}
}
5. NullPointerException:
NullPointerException is an unchecked exception that occurs when attempting to access or perform operations on an object reference that is null
.
String str = null;
try {
// Trying to invoke a method on a null reference
int length = str.length();
} catch (NullPointerException e) {
// Handle NullPointerException (e.g., provide a default value)
System.out.println("NullPointerException: " + e.getMessage());
}
User Defined Exception
A user-defined exception in programming is like creating your own special type of problem that your computer program can understand. It’s as if you’re saying, “If something very specific goes wrong, let’s handle it in a way that makes sense for our program.” So, it’s a way for the people writing the program to define and deal with issues in a customized manner, tailoring the solutions to fit the exact needs of their software.
Imagine you are building a program, and you want to handle certain situations in a special way. For example, let’s say you want to react differently when a user enters a negative number. In Java, there are standard ways to handle errors, but you can also create your own special way of handling errors, known as “user-defined exceptions.”
Code Example
// Custom exception class
class NegativeNumberException extends Exception {
// Constructor to create the exception with a message
public NegativeNumberException(String message) {
super(message);
}
}
// Example class using the custom exception
public class ExceptionHandlingExample {
// Method that might cause our custom exception
public static void processNumber(int number) throws NegativeNumberException {
if (number < 0) {
// Throw our custom exception with a message
throw new NegativeNumberException("Please enter a non-negative number");
}
// Continue with normal processing if the number is okay
}
// Main method to demonstrate the custom exception
public static void main(String[] args) {
int userNumber = -5;
try {
// Try to process the user's number
processNumber(userNumber);
} catch (NegativeNumberException e) {
// Handle our custom exception
System.out.println("NegativeNumberException: " + e.getMessage());
} finally {
// Code in this block runs no matter what
System.out.println("Finally block executed");
}
}
}
In this example:
- We created a special exception called
NegativeNumberException
. - When the user enters a negative number, we throw our custom exception.
- We use
try-catch
to catch and handle our special exception. - The
finally
block is like a safety net – it always runs, whether there was an issue or not.
So, user-defined exceptions allow you to make your program understand and respond to specific situations in a way that makes sense for your application. It’s like creating your own set of rules for how your program should behave when something unexpected happens.
Difference between User Defined Exception and System Defined Exception
Aspect | User-Defined Exception | System-Defined Exception |
---|---|---|
Definition | Created by programmers as per specific application needs. | Predefined in the programming language or runtime environment. |
Creation Process | Developers create a new class that extends Exception or its subclass. | Inherent in the language or libraries, not created by developers. |
Purpose | Tailored to handle application-specific scenarios. | Covers general or common error conditions. |
Flexibility | Offers flexibility in designing error handling strategies based on application logic. | Less flexible as it addresses general scenarios and may not align perfectly with specific application requirements. |
Example (Java) | java class MyException extends Exception { /* ... */ } | java try { /* ... */ } catch (NullPointerException e) { /* ... */ } |
Application Context | Best suited for handling unique situations within a specific program. | Appropriate for handling general errors that can occur across various programs. |
Extensibility | Can be extended and customized to meet evolving program requirements. | Generally static and does not change unless the programming language or libraries are updated. |
Usage | Employed when a program encounters specialized error conditions that are specific to its logic. | Used for handling common errors like null references, array out-of-bounds, file not found, etc. |
Examples (Java) | java throw new MyException("Custom error message"); | java throw new NullPointerException("Trying to access a method on a null object"); |
FAQ
Q1: What is exception handling?
Exception handling is a programming concept that deals with the detection, management, and resolution of unexpected or exceptional situations (errors) during the execution of a program.
Q2: Why do we need exception handling?
Exception handling is crucial for maintaining program stability. It allows developers to identify and gracefully handle unexpected situations, preventing abrupt program termination and providing a controlled way to respond to errors.
Q3: What is the difference between checked and unchecked exceptions?
Checked exceptions are required to be either caught (using try-catch) or declared in the method signature. Unchecked exceptions (RuntimeExceptions) are not mandatory to be handled explicitly.
Q4: How can I create my own exception in Java?
To create a custom exception in Java, you need to define a new class that extends either the Exception
class or one of its subclasses. This class represents your custom exception, and you can add your own methods or details to it.
Q5: What is the purpose of the ‘finally’ block in exception handling?
The ‘finally’ block is used to define a set of statements that will be executed whether an exception is thrown or not. It is often used for cleanup operations, like closing files or releasing resources, ensuring they are executed regardless of whether an exception occurs.