The Body Mass Index in assembly language with MIPS architecture is a widely used metric for assessing whether an individual’s weight is within a healthy range. This blog post explores the implementation of Body Mass Index in assembly language with MIPS architecture, calculating BMI based on user inputs—gender, age, weight (in kilograms), and height (in feet). It categorizes the user into four BMI classifications: underweight, normal, overweight, or obese, with a detailed explanation of the code for easy comprehension.
BMI classification using assembly language
1. Data Section
The data section of the program Body Mass Index in assembly language with MIPS architecture stores static data used throughout the execution.
This includes:
- Prompts for User Inputs: Strings such as “Enter your gender (M/F):” and “Enter your weight (kg):” are displayed to request input.
- Output Messages: Strings like “Your BMI is:” and classification messages (e.g., “You are classified as underweight.”) are displayed after processing inputs.
- Constants: These include conversion factors (e.g., 0.3048 for feet to meters) and BMI thresholds (18.5, 24.9, 29.9).
- Temporary Storage: Space for inputs like age, weight, and height, ensuring efficient memory utilization.
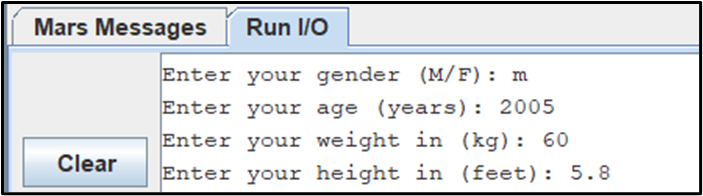
2. Text Section
The text section contains the program logic, implemented as a sequence of MIPS assembly instructions. Let’s explore this step-by-step:
a. Getting User Input
The program Body Mass Index in assembly language with MIPS uses system calls to interact with the user. It:
- Prompts the user to enter gender, age, weight, and height.
- Reads the inputs using syscall instructions and stores them in memory or registers for further use.
For instance:
- The
prompt_gender
string is displayed. - The user’s input is read and stored in a buffer for processing.
b. Converting Height to Meters
Since BMI calculations require height in meters, the program converts the user-provided height in feet using the formula:
This is achieved using a floating-point multiplication instruction. The converted height is stored for subsequent calculations.
c. Calculating BMI
The BMI is calculated using the formula:
The program:
- Squares the height in meters.
- Divides the weight by the squared height using floating-point instructions.
- Stores the result as the user’s BMI.
d. Displaying BMI
The program displays the calculated BMI using a syscall. This provides the user with immediate feedback on their result.
e. If-else conditions in MIPS assembly language
To categorize BMI, the program compares the calculated value against predefined thresholds:
- Underweight: BMI < 18.5
- Normal: 18.5 ≤ BMI < 24.9
- Overweight: 25.0 ≤ BMI < 29.9
- Obese: BMI ≥ 30.0
Using conditional branching (c.lt.s
, c.le.s
), the program determines the appropriate category and displays the corresponding message.
f. Displaying BMI Classification Table
To enhance user understanding, the program outputs a BMI classification table showing all categories and their thresholds. This provides additional context to the user.
g. Program Termination
After displaying results, the program gracefully ends using a syscall. This ensures a clean exit and resource release.
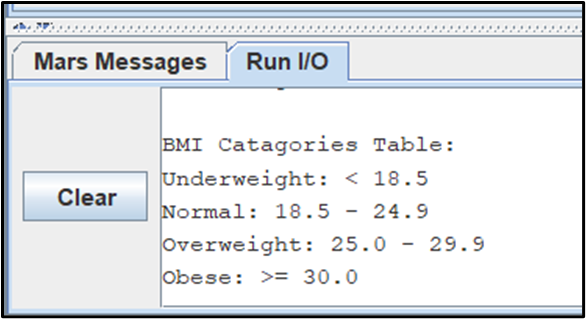
Basics concepts of assembly language with MIPS
- System Calls (Syscalls):
- Used for input/output operations.
- Examples include displaying strings, reading integers, and reading floating-point numbers.
- Floating-Point Arithmetic:
- Essential for accurate calculations.
- Operations like multiplication, division, and comparison are used extensively.
- Branching and Control Flow:
- The program uses conditional instructions to navigate through BMI categories.
- For example,
bc1t
(branch on condition true) directs the flow based on BMI thresholds.
- Memory Management:
- Inputs and intermediate values are stored efficiently in memory or registers.
- Temporary storage ensures that calculations do not overwrite user-provided data.
Understanding the Role of Registers in MIPS Assembly
Holds the address to return to after a function call. While not directly used in this linear program, it’s vital in subroutine-based assembly programming.
$v0
registers:
Purpose: Holds the syscall code for various operations such as printing strings, reading inputs, or exiting the program.
Example:
- Before printing a string,
$v0
is set to4
. - Before reading an integer,
$v0
is set to5
.
$a0
register:
Purpose: Points to the memory address of a string to be displayed or holds the first argument for a syscall.
Example:
- Before displaying the “Enter your gender” prompt,
$a0
is loaded with the address ofprompt_gender
.
$a1
register:
Purpose: Holds the second argument for syscalls, typically used when reading a string input.
Example:
$a1
is loaded with the size of the input buffer (2
bytes) when reading the gender input.
$f0
:
Purpose: A floating-point register used for holding floating-point inputs or intermediate results.
Example:
- When the user enters their weight or height, it’s initially stored in
$f0
.
$f12
, $f13
, $f14
, $f15
, $f16
, $f17
, $f18
register:
Purpose: Floating-point registers used for arithmetic operations and comparisons.
Detailed Usage:
$f12
: Holds the weight during the BMI calculation.$f13
: Stores the converted height in meters.$f14
: Temporarily holds the squared height during the BMI calculation.$f15
: Stores the final BMI value.$f16
,$f17
,$f18
: Store the BMI thresholds for comparison.
$t0
, $t1
, etc. (Temporary Registers):
- Not explicitly used in the provided code but can be employed for temporary integer operations if required.
$sp
(Stack Pointer):
- Not directly manipulated in the code, but essential in MIPS assembly for managing function calls or local variables if implemented.
$ra
(Return Address):
- Holds the address to return to after a function call. While not directly used in this linear program, it’s vital in subroutine-based assembly programming.
Real-world applications of MIPS assembly programming
To make this program more understandable, here’s an example of its execution:
- Input Stage:
- The user enters the following:
- Gender:
M
- Age:
25
- Weight:
70 kg
- Height:
5.8 feet
- Gender:
- The user enters the following:
- Conversion:
- Height in meters =
5.8 × 0.3048 = 1.76864 m
- Height in meters =
- BMI Calculation:
- BMI =
70 / (1.76864^2) = 22.3
- BMI =
- Classification:
- Based on the BMI value of
22.3
, the program classifies the user as having a “Normal” weight.
- Based on the BMI value of
- Output:
- BMI:
22.3
- Classification: “Your weight is in the normal range.”
- Classification Table: Displayed for reference.
- BMI:
Conclusion of BMI classification using assembly language
This MIPS assembly program provides a practical example of low-level programming to solve real-world problems like BMI calculation. By breaking down the logic and using clear instructions, it bridges the gap between user inputs and meaningful outputs.
Whether you are a beginner exploring assembly language or an experienced programmer seeking insights, this program serves as an excellent learning opportunity. Through hands-on implementation, you’ll develop a deeper understanding of floating-point arithmetic, control flow, and efficient memory usage in MIPS assembly.
Source Code of Body Mass Index in assembly language with MIPS
.data
# Prompts for user inputs
prompt_gender: .asciiz "Enter your gender (M/F): "
prompt_age: .asciiz "\nEnter your age (years): "
prompt_weight: .asciiz "Enter your weight in (kg): "
prompt_height: .asciiz "Enter your height in (feet): "
# Output messages
output_bmi: .asciiz "\nYour BMI is: "
category_underweight: .asciiz "You are classified as underweight.\n"
category_normal: .asciiz "Your weight is in the normal range.\n"
category_overweight: .asciiz "You are classified as overweight.\n"
category_obese: .asciiz "You are classified as obese.\n"
newline: .asciiz "\n"
category_table: .asciiz "\nBMI Catagories Table:\nUnderweight: < 18.5\nNormal: 18.5 - 24.9\nOverweight: 25.0 - 29.9\nObese: >= 30.0\n"
# Constants and values
conversion_factor: .float 0.3048
category_18_5: .float 18.5
category_24_9: .float 24.9
category_29_9: .float 29.9
# Temporary storage
buffer_gender: .space 2
age: .word 0
weight: .float 0.0
height: .float 0.0
.text
.globl main
main:
# Ask for gender
li $v0, 4
la $a0, prompt_gender
syscall
#Read gender input
li $v0, 8
la $a0,buffer_gender
li $a1, 2
syscall
# Ask for age
li $v0, 4
la $a0, prompt_age
syscall
# Get age input
li $v0, 5
syscall
sw $v0, age
# Ask for weight
li $v0, 4
la $a0, prompt_weight
syscall
# Get weight input
li $v0, 6
syscall
s.s $f0, weight
# Ask for height
li $v0, 4
la $a0, prompt_height
syscall
# Get height input in feet
li $v0, 6
syscall
s.s $f0, height
# Convert height to meters
l.s $f13, height
l.s $f16, conversion_factor
mul.s $f13, $f13, $f16
# Load weight for BMI calculation
l.s $f12, weight
# Calculate BMI (BMI = weight / (height^2))
mul.s $f14, $f13, $f13
div.s $f15, $f12, $f14
# Display BMI value
li $v0, 4
la $a0, output_bmi
syscall
li $v0, 2
mov.s $f12, $f15
syscall
# Add a newline for formatting
li $v0, 4
la $a0, newline
syscall
# Determine BMI category
l.s $f16, category_18_5
l.s $f17, category_24_9
l.s $f18, category_29_9
c.lt.s $f15, $f16
bc1t underweight_case
c.le.s $f15, $f17
bc1t normal_case
c.le.s $f15, $f18
bc1t overweight_case
# Case for obese category
la $a0, category_obese
j display_category
underweight_case:
la $a0, category_underweight
j display_category
normal_case:
la $a0, category_normal
j display_category
overweight_case:
la $a0, category_overweight
display_category:
li $v0, 4
syscall
# Show the BMI classification table
li $v0, 4
la $a0, category_table
syscall
# End the program
li $v0, 10
syscall