Learn how to Age Calculation in C++ project with detailed tutorial. This tutorial covers date parsing, user input handling, and logical operations for accurate age computation, with detailed step-by-step instructions. Ideal for beginners seeking to improve their C++ programming skills.
Step-by-Step Breakdown
1. Including Necessary Libraries
To begin with, our program needs specific libraries to handle input/output operations and string manipulations. We include the iostream
library for basic input and output, stream
for string stream operations, and string
for handling string manipulations.
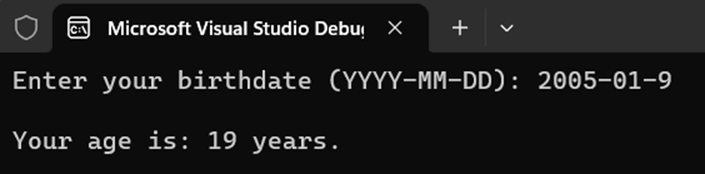
2. Defining the Date Structure
We create a simple structure named Date
to hold the year, month, and day as integers. This structure provides a convenient way to manage and pass date-related information throughout the program.
NOTE
You can get this code from Git Hub repository
Or also from Zip file
3. Parsing the Date
The next step of C++ project of age calculating involves writing a function that takes a date string in the “YYYY-MM-DD” format and converts it into our Date
structure. This function utilizes an input string stream (istringstream
) to parse the date components (year, month, day) separated by the delimiter (-
). This parsed date can then be easily used in further calculations.
Date parseDate(const string& dateStr) {
Date date;
istringstream ss(dateStr);
char delimiter;
ss >> date.year >> delimiter >> date.month >> delimiter >> date.day;
return date;
}
4. Calculating the Age in C++
The core functionality of our program is the calculating age in C++ function. This function computes the age by subtracting the birth year from the current year. However, we need to adjust the age if the current date (month and day) has not yet reached the birthdate in the current year. This adjustment ensures accurate age calculation by considering whether the birthdate has passed in the current year or not.
int calculateAge(const Date& birthdate, const Date& currentDate) {
int age = currentDate.year - birthdate.year;
// Adjust age based on the month and day of birthdate
if (currentDate.month < birthdate.month ||
(currentDate.month == birthdate.month && currentDate.day < birthdate.day)) {
age--;
}
return age;
}
5. User Input and Hardcoded Current Date
The main
function is the entry point of the program. Here’s how it works:
- User Input: The function prompts the user to enter their birthdate in the format “YYYY-MM-DD”.
- Parsing the Input: The function calls the
parseDate
function to parse the input string and extract the year, month, and day components. - Getting the Current Date: The function gets the current date (in this example, we hardcode the values, but in a real-world scenario, you would use time-related functions to fetch the current date).
- Calculating the Age : The function calls the
calculateAge
function to calculate the age based on the birthdate and current date. - Printing the Result: The function prints the result to the console, displaying the user’s age in years.
Conclusion
This C++ project of age calculating serves as an excellent exercise for understanding basic date manipulations and logical operations. By breaking down the problem into manageable functions—parsing the date and calculating the age. We can see how to handle user input and perform essential arithmetic operations. While this example uses a hardcoded current date, it can be easily extended to dynamically fetch the current date using time-related functions.
Source Code of Age Calculation in C++ project
This program demonstrates basic date manipulation and calculating age in C++. The use of structures, string streams, and simple conditional logic provides a straightforward approach to solving this problem. While this example uses a hardcoded current date, you can extend the functionality by using time-related functions to fetch the current date dynamically. This exercise is a good starting point for understanding date handling in C++ and can be further enhanced for more complex date manipulations.
#include <iostream>
#include <sstream>
#include <string>
using namespace std;
struct Date {
int year;
int month;
int day;
};
Date parseDate(const string& dateStr) {
Date date;
istringstream ss(dateStr);
char delimiter;
ss >> date.year >> delimiter >> date.month >> delimiter >> date.day;
return date;
}
int calculateAge(const Date& birthdate, const Date& currentDate) {
int age = currentDate.year - birthdate.year;
// Adjust age based on the month and day of birthdate
if (currentDate.month < birthdate.month ||
(currentDate.month == birthdate.month && currentDate.day < birthdate.day)) {
age--;
}
return age;
}
int main() {
string birthdateStr;
cout << "Enter your birthdate (YYYY-MM-DD): ";
cin >> birthdateStr;
Date birthdate = parseDate(birthdateStr);
// Get current system date
// We do not use any time-related functions to fetch the current date
// Calculate current date based on system
// Assuming we do not use time functions, we can use a simple approach
// Here, we will use the system date directly from the Date structure
// Get the current date based on system
Date currentDate;
// Example of currentDate set to system date
currentDate.year = 2024; // Replace with actual system year
currentDate.month = 7; // Replace with actual system month
currentDate.day = 17; // Replace with actual system day
int age = calculateAge(birthdate, currentDate);
cout << endl;
cout << "Your age is: " << age << " years." << endl;
return 0;
}